Are you aiming to launch powerful, user-friendly mobile applications? Backbone.js could be just the tool to make that happen. In an era where consumer expectations for mobile experiences are continually rising, adopting Backbone can give you a competitive edge.
This JavaScript framework was designed to simplify front-end development, organizing an app's visual and interactive parts, by structuring code with “models” and “views.” If you build applications with rich user interactions, this framework can help you sync data and update the user interface in real time, improving the overall user experience.
Thanks to its strong emphasis on efficient code management and user interactions, Backbone has become a great choice for those looking to optimize performance and cut costs.
Let's see how Backbone.js can fit into your strategy.
Introduction to Backbone.js
What is Backbone.js?
Backbone.js, created in 2010 by Jeremy Ashkenas (the developer behind CoffeeScript and Underscore.js), is a JavaScript framework designed to make front-end development easier and more organized. It's an open-source library, which means it's free to use, with more than 100 extensions available for added functionality.
At a time when web development was becoming increasingly complex, Backbone emerged as a solution to simplify and structure applications, making them more maintainable and scalable over time.
Backbone’s core components, like Models, Views, Collections, and Routers, work together to create a flexible “backbone” for data management and user interface updates. Backbone.js models handle data logic, Views display information and respond to user actions, and Routers manage navigation. That is why, when applications are broken down this way, Backbone reduces the amount of code required and makes everything more modular and reusable.
Advantages of Using Backbone.js
Lightweight and Efficient: At only 7.6KB, Backbone.js is fast and lightweight, keeping applications agile and responsive.
Free and Open-Source: With a large community, developers have access to resources, plugins, and support, helpful for both quick fixes and new features.
Organized Structure: Backbone provides essential components like models, views, and controllers, keeping code clean and organized.
Automatic HTML Updates: HTML updates automatically based on data changes, making it easier to build applications without extra coding.
RESTful API Compatibility: Works fluidly with RESTful APIs, ensuring frictionless communication with backend systems.
Reduced Maintenance: For businesses, Backbone’s structure translates to easier maintenance, faster updates, and a more reliable and stable product.
Scalability: Developers can build small or complex applications that can scale as needed, creating long-term value for businesses.
Getting Started with Backbone.js
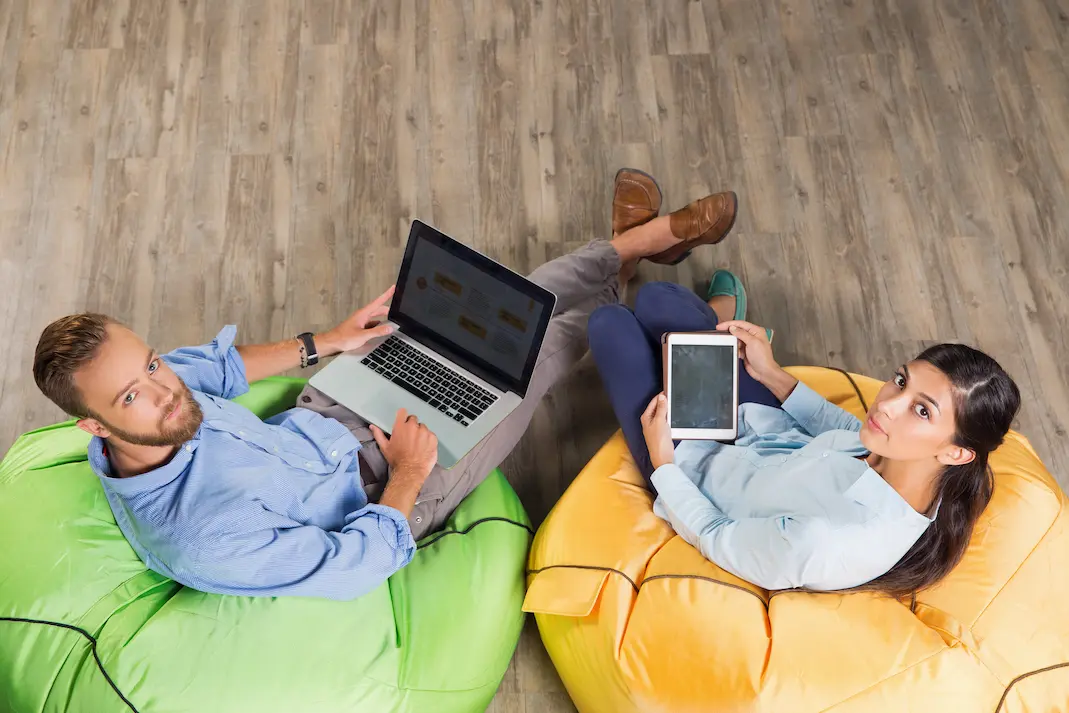
Setting Up Backbone.js
Thankfully, Backbone is known for its simplicity and lightweight structure, making installation quick and straightforward. You can add Backbone to your project in two main ways. One option is to download it directly from the official website and include it in your project’s code files. Alternatively, you can install it using a package manager like npm by typing npm install backbone
into your command line.
Backbone requires both Underscore.js and jQuery (or a similar library) to function properly, so make sure these are also included. In fact, Backbone.js works perfectly with jQuery, which is often used for DOM manipulation, and Underscore.js. For example, you can create Backbone.js models to handle data and sync it with your backend, then use views to manage the UI elements and display the app’s data.
Creating Models and Views
Creating models and views in Backbone.js is a straightforward process that brings structure and clarity to the front-end development.
In Backbone, a model represents the data and business logic of an application. For example, if you’re building an app to manage tasks, each task would be a model, containing details like the task name, deadline, and status.
On the other hand, views are all about presentation. They control what users see and how they interact with the app, presenting data and handling interactions, like updating a task’s status when it’s completed.
Once a model changes, the view can automatically update, saving developers time and making the app more responsive for users. Together, Backbone.js models and views create a valuable, organized framework for front-end projects.
Working with Collections
Collections are essentially groups of models, that are key when you're working with multiple pieces of data that share a similar structure or purpose. They are great for organizing the data into logical groups, like managing a list of users, products, or posts.
To work with collections in Backbone, you have to start by creating a new collection instance, which is tied to a model. You can add, remove, or fetch models as needed, all while keeping things organized.
Overall, collections are great for organizing data in the front-end app, ensuring that the code stays clean and manageable as the project continues to evolve. So, whether you're migrating Backbone or starting from scratch, collections make handling complex data structures more intuitive and scalable.
Key Components of Backbone.js
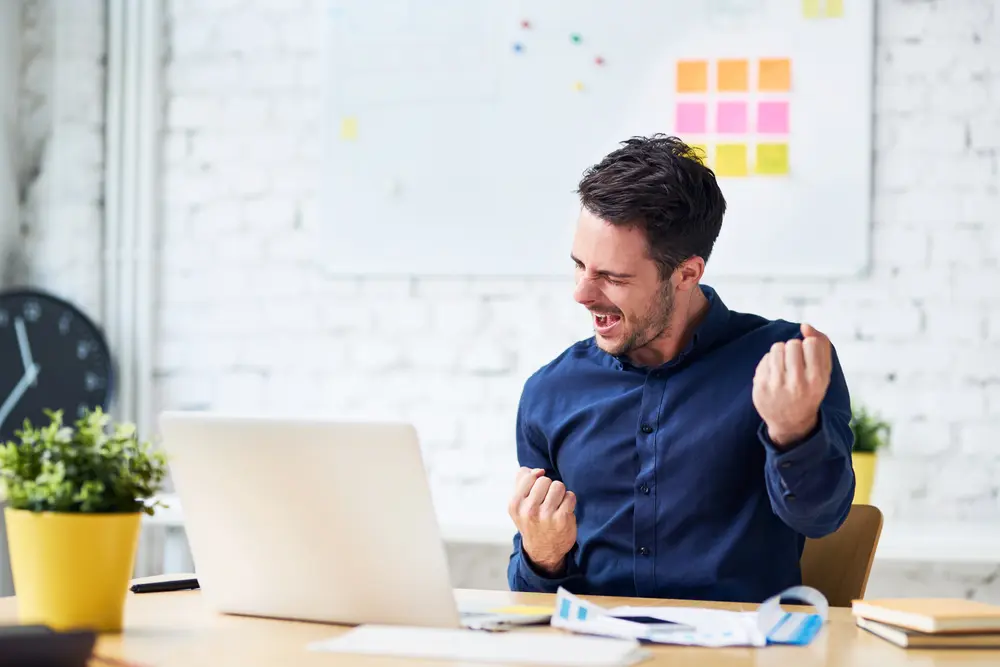
Understanding Backbone Events
Backbone.js events allow different parts of an application to communicate with each other by triggering and listening for specific actions. This means that they allow different parts of the app, like models, views, and controllers, to communicate without being tightly coupled. When something changes, like a model being updated, an event is triggered, which other components can listen for and respond to.
Exploring Backbone Models
Models are an essential part of the JavaScript framework, acting as the foundation for an app's data. They manage attributes and handle logic, ensuring data is organized and synced. Each model represents a single entity, like a user or product, and stores its attributes as key-value pairs.
Backbone.js models can also include custom methods to handle specific behaviors. They interact with views to update the user interface and sync with the server, ensuring data stays consistent. This structure makes it easier to organize and scale an application.
Utilizing Backbone Collections
In Backbone.js, collections are essential for managing groups of related models. For example, a collection can hold multiple models, making it easier to perform bulk operations like adding, removing, or sorting them. What's more, these elements offer built-in methods for fetching data from the server and binding changes to the view, which simplifies data management. Lastly, when migrating Backbone, collections play a key role in maintaining a clean, efficient, and scalable code structure.
Implementing Backbone Router
The Backbone.js router is essential for managing client-side navigation in single-page applications. It maps URL fragments to specific actions, triggering the display of corresponding views. When a user interacts with a URL, the router listens for changes and routes them to the right part of the application, making it easy to manage and navigate different parts of the app.
Managing History with Backbone History
Backbone.js History is another built-in feature that helps manage navigation in single-page applications. It functions as a global router that tracks the history of an app’s navigation. To use it, you create a router class and call Backbone.history.start()
. This starts monitoring URL changes and triggers routes based on them, allowing the app to update the view without refreshing the page.
Performing Data Sync with Backbone.sync
The Backbone.sync
method has a vital role in syncing data between the client-side models and the server. It manages CRUD operations (Create, Read, Update, Delete) for Backbone.js models, which allows to easily send and receive data in formats like JSON. When a sync is called, it communicates with the server's API, updating the app's data automatically and ensuring models stay in sync with the server.
Rendering Views with Backbone.View
Using Backbone.View
, you can bind HTML elements to the JavaScript models, keeping the UI in sync with the data. When the model changes, the view automatically updates, making it easy to manage dynamic content. Rendering a view involves defining how the data should be displayed and then using the render()
method to update the view whenever the model changes.
Advanced Features and Integration
Backbone.js also provides extensions like its localStorage adapter, which facilitates data persistence on the client side. Additionally, it supports direct integration with other libraries and frameworks, enabling frictionless transitions when migrating to newer technologies. These advanced features make it easier for developers to scale applications while maintaining outstanding performance and a clean, organized codebase.
Integrating API with Backbone.js
Connecting an API with Backbone is simpler than it might seem, and it brings a lot of value to an app. By linking Backbone.js models to the RESTful API, the app can pull in data or save updates in real-time, all while keeping things organized. It’s a simple way to standardize the development process and improve performance without complicating things.
Implementing View Rendering Techniques
With Backbone.js view rendering techniques, you can control when specific parts of an app's interface update, making it easier to display data efficiently and keeping the user interface responsive. That way, you can control exactly when and how different parts of the screen update, so the code stays clean and easy to work with, even as the app grows in complexity.
Working with Routes and URLs
Routes and URLs help map user interactions to specific views within an app. When you define routes, you are essentially telling Backbone how to handle different URL patterns and what view to display for each. That way, when a user clicks a link or enters a URL, Backbone loads the correct view without refreshing the page. In a nutshell, this process helps create a more dynamic, app-like experience, ensuring the app feels fast and responsive.
Utility Functions in Backbone.js
Utility functions in Backbone.js are like time-savers for developers. They simplify common tasks such as managing events, handling models, and updating views. Instead of writing custom code from scratch, these built-in tools allow you to quickly implement standard features and help you build more efficiently and with fewer headaches, letting you focus on the creative parts of the project.
Real-world Examples and Case Studies
Backbone.js has made a significant impact in the world of front-end development, helping companies across the world build efficient, scalable, and dynamic web applications.
Right now, Backbone is used in 36 countries, with the United States leading the way. More than 400 companies across the US rely on it, while India and the United Kingdom follow with 84 and 52 companies, respectively.
In technology, companies like Atlassian and MongoDB rely on Backbone for scalable apps. It's also popular in project management with platforms like ClickUp and Monday.com, where interactivity is key.
In e-commerce, Backbone powers fast, responsive websites like WP Engine and Demandware. It’s also used in media, education, and healthcare to build dynamic, real-time applications.
Its flexibility and speed make it a great fit for any project that needs an interactive user experience, and this global reach shows how Backbone.js has found its place in companies of all sizes and industries.
So when is Backbone the right fit? Here are a few common scenarios:
Building single-page apps with minimal dependencies
Backbone.js works great when you don’t need too many extra libraries or tools.
Heavy lifting in the backend
If your backend is doing most of the work, Backbone can handle the front end without getting in the way.
Fast, functional web apps
If you need to quickly develop an interactive web app without compromising on quality or performance, Backbone.js has you covered.
It’s no surprise that Backbone is used by companies in tech, education, and even entertainment. The framework’s ability to simplify development processes, reduce complexity, and improve performance makes it a valuable asset for teams looking to build outstanding, scalable applications.
Overall, it’s a versatile tool that can help you deliver a great product without a lot of overhead. So, whether you’re building something simple or more complex, Backbone makes the job easier.
Curious about Java? Find out how the top companies that use Java are innovating and scaling their businesses—read now!
Comparative Analysis
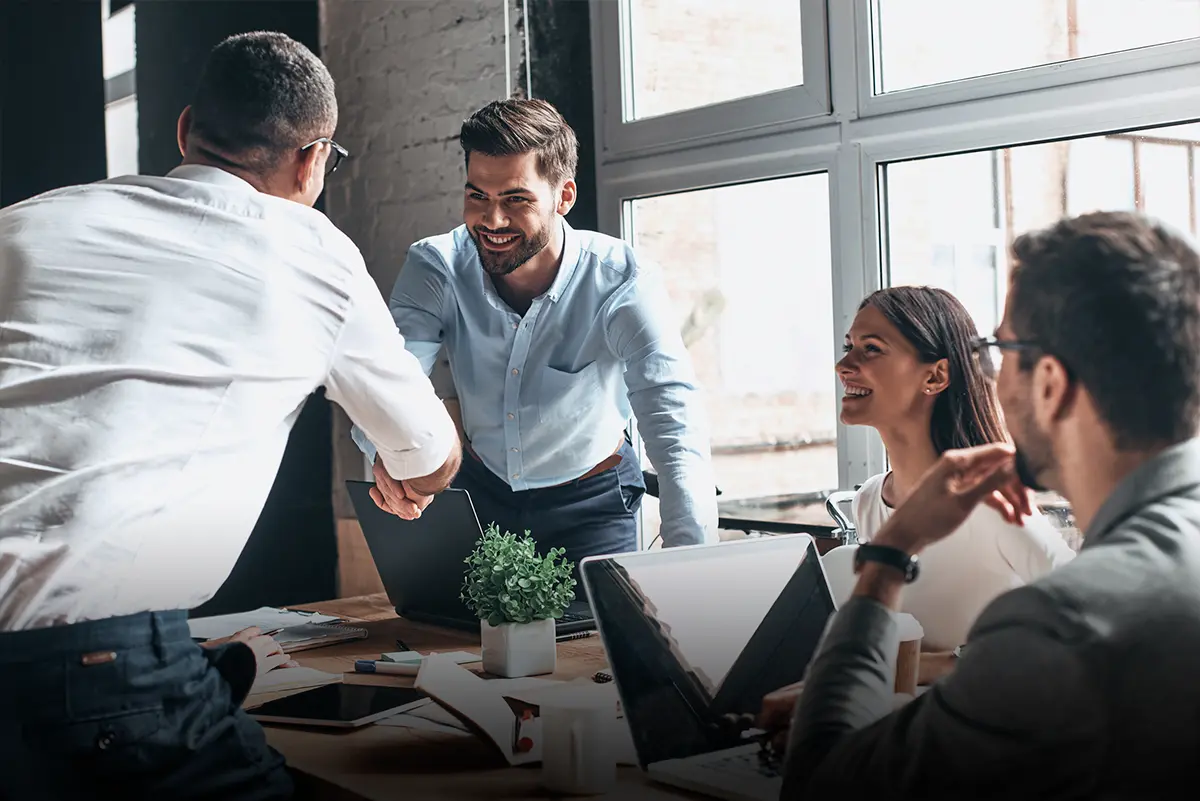
React vs. Backbone.js: Choosing the Right Framework
When choosing between React and Backbone, it’s important to consider the needs of the project and each framework's strengths.
React is designed to help developers build interactive, dynamic user interfaces, especially for complex applications with lots of moving parts. A key use case for React is developing interactive dashboards, like those for analytics or project management tools, where real-time data updates (such as graphs or stats) are crucial.
Backbone.js, in contrast, is a lightweight framework that offers a more minimalistic approach. It’s structured around models, views, and collections, providing basic tools to organize the JavaScript code. While it's not as feature-rich as React, Backbone is ideal for simpler applications or when you prefer more flexibility with fewer constraints.
In short, React excels in handling complex, data-driven UIs, while Backbone.js is better for smaller, less interactive apps where simplicity and flexibility are key. Both frameworks have their place, depending on the scope and complexity of the project.
Pros and Cons of React.js
React Pros
React is super popular for a reason. It’s fast, thanks to its virtual DOM, which makes rendering smooth even in complex apps. The component-based design allows for easy code reuse, saving time on big projects. Plus, with ReactNative, you can even build mobile apps. And a big bonus is the huge community that’s always ready to help.
React Cons
However, React’s learning curve can be a bit steep, especially if you're new to concepts like JSX. Also, when your app gets big, managing the state can get a little complicated, so you might need extra tools like Redux.
Read this to discover The Java Virtual Machine (JVM) and learn how its robust runtime environment drives modern software development today!
Pros and Cons of Backbone.js
Backbone.js Pros
Backbone is lightweight and simple, making it a great option for smaller, straightforward apps. It’s easy to understand, with built-in support for models, views, and controllers, making it great for quick setups.
Backbone.js Cons
That said, Backbone can feel a bit basic compared to newer frameworks. For larger apps, it might require a lot of extra code and libraries to manage things efficiently, which can slow things down.
Your Backbone.js Project Deserves Expert Developers
Backbone offers a straightforward, flexible framework ideal for building dynamic web apps, especially when you are focused on simplifying the code and structure. However, as with any technology, the key to success lies in having experts who truly understand it. If you’re looking to implement Backbone.js in your project or migrate to it, having experienced professionals on your team makes all the difference.
At Jalasoft, we specialize in connecting you with skilled professionals who know Backbone.js inside and out. Our team can help you optimize your front-end development projects, ensuring that you're not just using the framework but using it in the best way possible. Contact us today, and let’s work together to ensure your next project is in the hands of real professionals.