Even the most skilled developers can make mistakes. As the legendary programmer Sam Redwine once said, "If debugging is the process of removing bugs, then programming must be the process of putting them in." Though humorous, this truth highlights the inevitable battle against errors in code.
These errors can cause programs to malfunction, crash, or produce unexpected results when programming. This article cuts through the technical jargon and provides the information you need to deal with the most common errors in software development.
We'll explore the common pitfalls and errors when programming, giving you a clear understanding of what might be blocking your development team. More importantly, we'll discuss practical strategies for efficiently identifying and fixing the different types of errors.
So, let's turn those bugs into opportunities for growth!
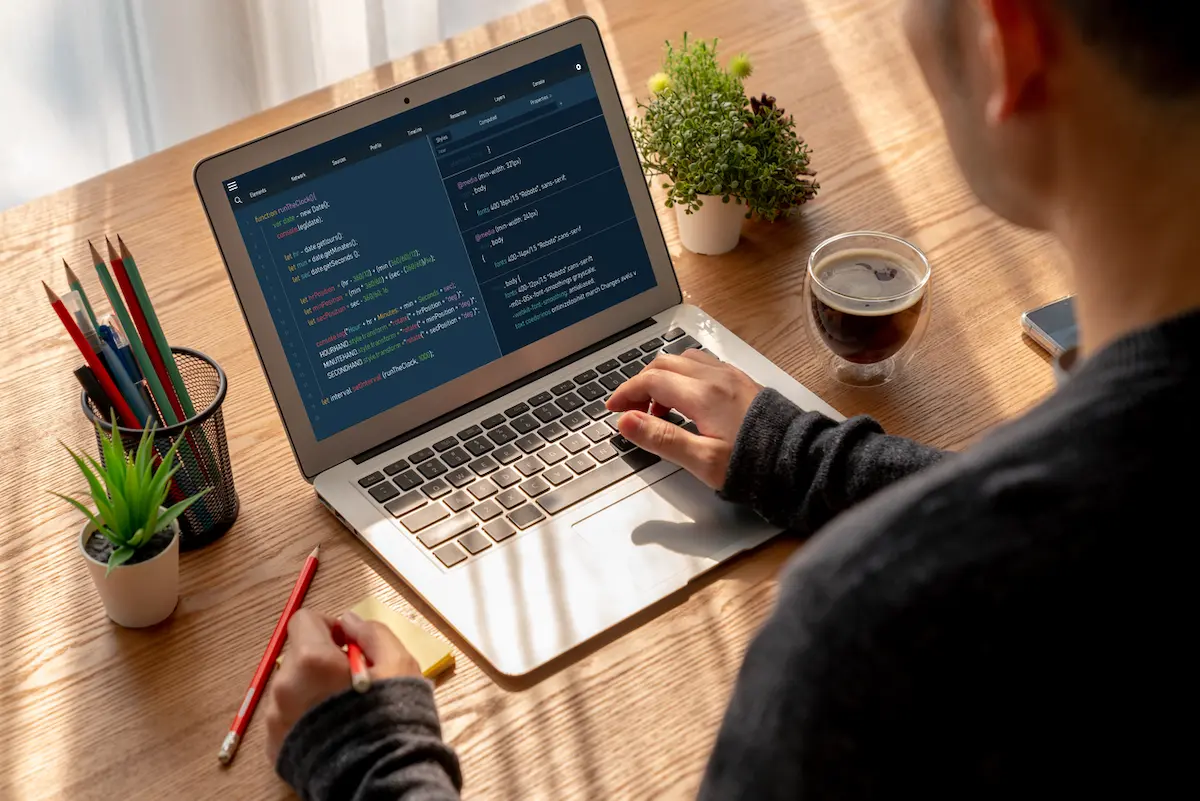
Syntax Errors
Understanding Syntax Errors in Coding
A syntax error is one of the most common errors when programming. It’s a fundamental mistake that prevents a program from running, occurring when the code violates language rules. For example, typos, missing semicolons, or mismatched brackets. However, though these errors can be easily identified and fixed when programming, they will also slow development if not caught early.
Utilizing Highlighting Tools to Detect a Syntax Error
Syntax highlighting uses color coding to differentiate keywords, functions, variables, and other elements, helping developers quickly identify inconsistencies and potential errors. For example, a missing semicolon or a misspelled keyword might be highlighted in red, alerting the development team about a potential syntax error.
Suppose we have this Python code snippet with a syntax error:
def greet(name): print("Hello, " + name) greet("Alice"
The code above has a missing closing parenthesis in the greet function call.
The greet("Alice" line may be highlighted differently, indicating an incomplete statement.
The IDE might underline the greet function call or use a different color to signal the syntax error.
And correct the code:
def greet(name): print("Hello, " + name)
greet("Alice")
By adding the closing parenthesis, the syntax error is resolved.
Logic Errors
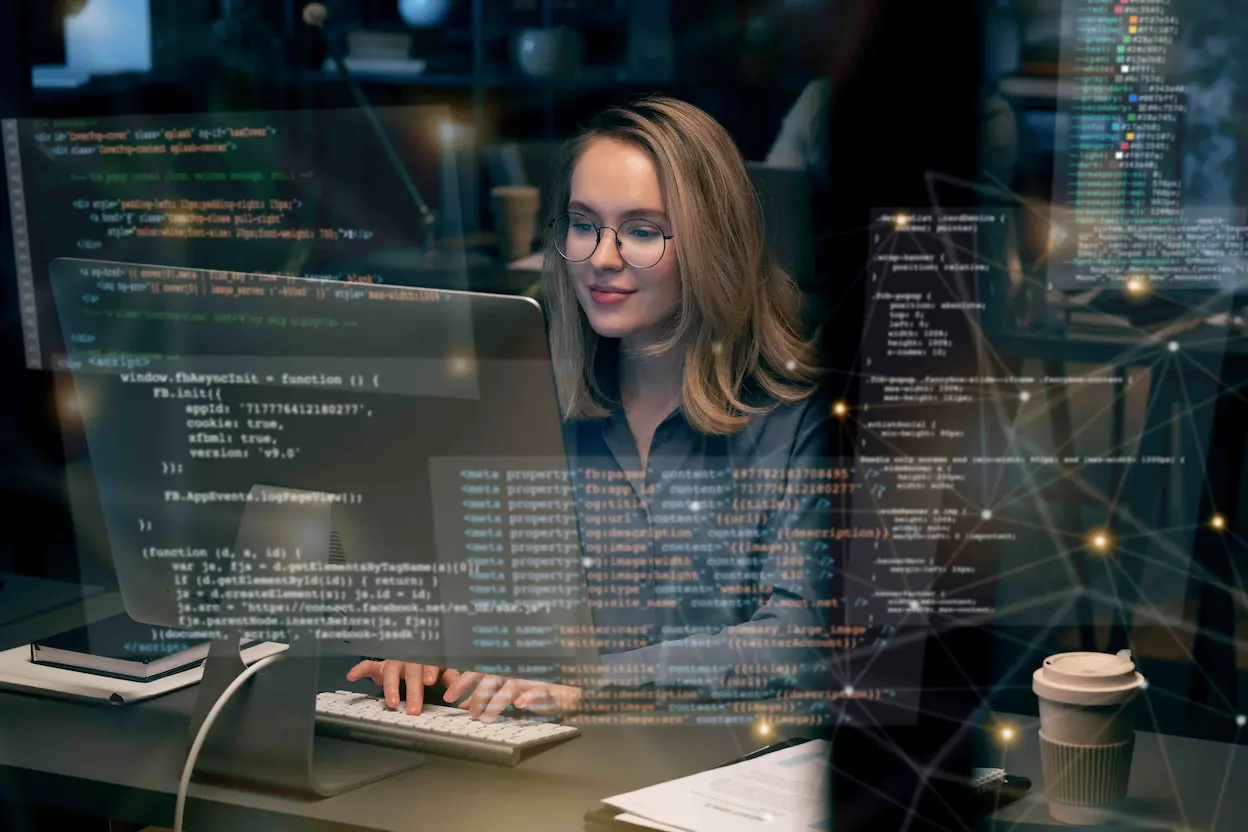
Recognizing a Logic Error and Their Impact on Code
Even a code that runs smoothly can have hidden flaws. A logic error, or logical error, occurs when the code's internal reasoning is faulty, leading to incorrect results. Unlike syntax or a runtime error, logic errors are often unnoticed until they impact functionality.
Strategies for Debugging a Logic Error
A logic error can be like a puzzle. That’s why, to identify the source of the problem, experienced developers use a systematic approach:
They start by reviewing the code's steps to review the logic and identify any flaws.
Devs create simplified scenarios, testing with sample data to isolate where the errors occur.
They compare expected results with actual outputs, highlighting where the logic deviates from the desired outcome.
Implementing Unit Testing to Identify a Logic Error
Unit testing focuses on individual functions, ensuring tasks perform correctly. This is especially helpful in detecting a logical error because by testing smaller code units, developers can prevent errors from becoming more significant problems later in development.
Runtime Errors
What is a Runtime Error and its Causes
These errors occur (as its name suggests) when a program bumps into an issue during the execution, even when the code is grammatically correct. Among the leading causes of a runtime error, we can find code bugs, resource limitations, or even user actions like typos.
Debugging a Runtime Error
A runtime error is the most similar to an unexpected roadblock. However, with the right tools, developers can proactively tackle them. These practices include examining code logs, using debuggers to crawl through the code line-by-line, or replicating the error with test data.
Best Practices for Handling Runtime Errors in Code
While a runtime error can almost never be entirely eliminated, proactive coding practices can minimize its impact. These include incorporating error-handling routines capable of managing issues and providing informative messages, which helps developers identify the root cause and guarantees the software remains stable.
Semantic Errors
Exploring Semantic Errors and How They Affect Code Execution
A semantic error occurs when the code structure is correct but uses the wrong elements or performs operations in the wrong order. These errors can be tricky to identify, as the code may run, but it won't produce the intended results.
Using Code Analysis Tools to Detect a Semantic Error
A semantic error is tricky to spot manually, which is why analysis tools can be beneficial. They can scan the code for potential issues, including using a variable that hasn't been defined or performing an illogical operation. Some common tools to detect a semantic error include SonarQube, ReSharper, Infer, and PMD.
Resolving a Semantic Error Through Refactoring
Refactoring helps developers fix a semantic error within the code, restructuring it and improving its clarity without changing its functionality. Therefore, by reorganizing the code, devs eliminate hidden semantic errors, leading to more accurate and reliable software.
Learn about the Benefits of having a Nearshore Software Development Partner with ISO 27001 Certification here
For companies looking to enhance software quality and optimize debugging processes, one effective approach is to collaborate with developers from Latin America who bring strong analytical skills and experience in debugging complex issues. Latin American devs are known for their problem-solving capabilities, ensuring efficient code that minimizes errors and enhances software performance.
Compilation Errors
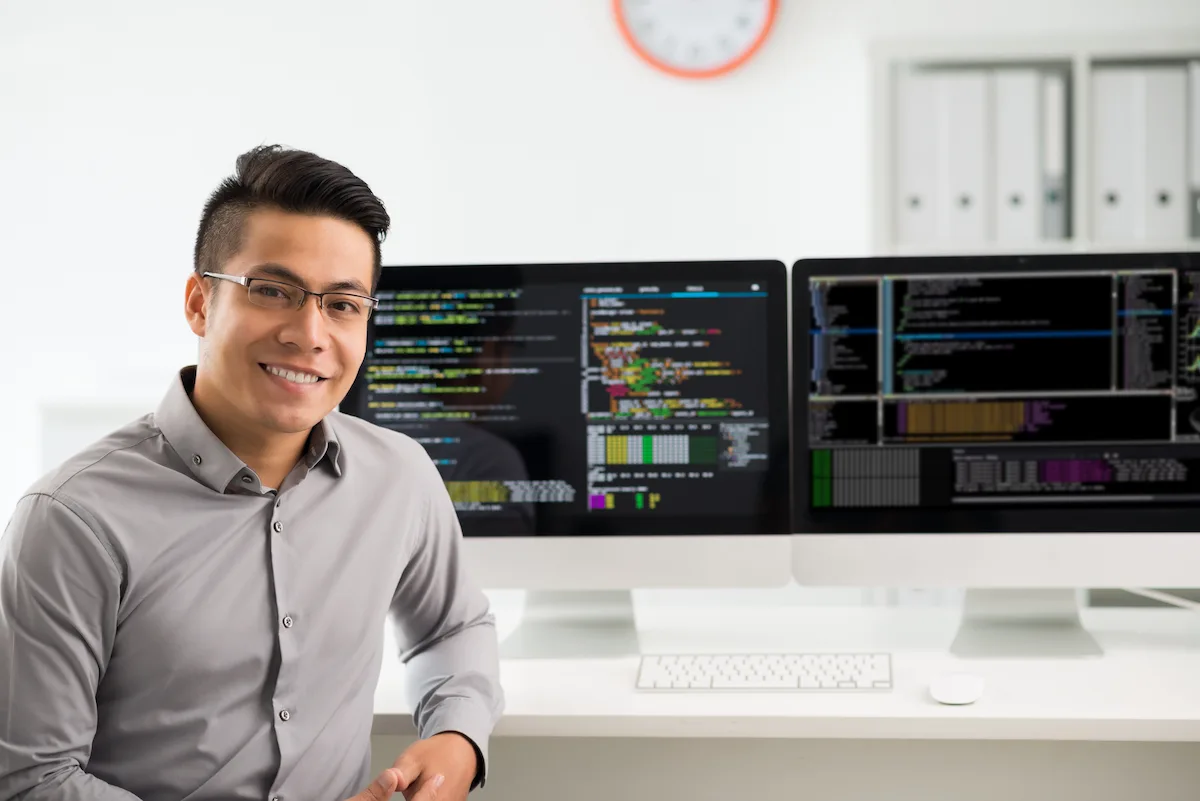
What is a Compilation Error?
Compilation errors occur before the code even runs when a program called the compiler can't understand the instructions due to typos or syntax issues. Though these are common mistakes, errors can also arise from missing libraries or limitations of the compiler itself.
Tips for Preventing Compilation Errors During Development
Familiarize yourself with the language's syntax. For example, pay attention to punctuation, keywords, and structure of elements.
Use smart text editors or Integrated Development Environments (IDEs) that highlight syntax errors and offer error checking as you write.
Compile your code frequently. This catches errors proactively, making them easier to fix.
Read error messages; don't skip them. They can show you the location and nature of the problem and act as a guide to resolving it.
Time Limit Exceeded Errors
Understanding Time Limit Exceeded Errors
"Time Limit Exceeded" (TLE) errors occur when the code takes too long to process the information. This often happens, for example, due to inefficient algorithms or excessive loops. So, while the code might work for smaller tasks, it can become sluggish and exceed the allotted time for larger datasets.
Strategies to Optimize Code Performance and Avoid Time Limit Errors
Streamline your code: Focus on efficiency. For example, look for ways to simplify loops or calculations, especially when dealing with large amounts of data.
Choose the right algorithm: The approach you take will significantly impact speed and reduce errors when programming. Explore different algorithms to find the one that solves your problem most efficiently.
Learn from others: Online resources and code comments can offer valuable insights when these errors occur. Maximize these resources to identify potential improvements.
Best Practices for Preventing Common Coding Errors
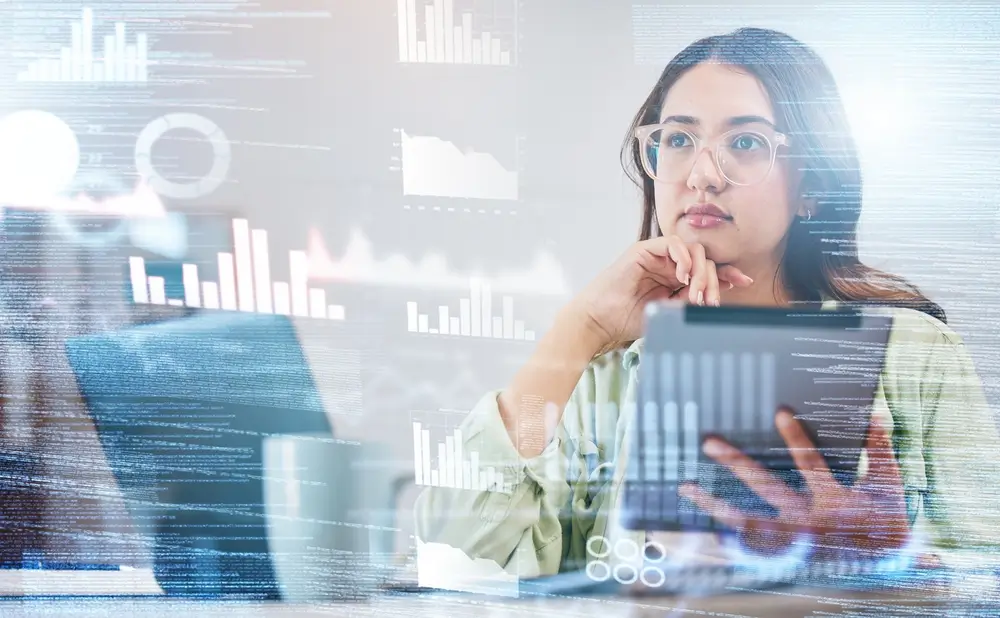
Writing Clean and Readable Code to Minimize Errors
Like a cluttered desk where everything seems hard to find, the same happens with code. A well-organized code uses clear variable names and includes comments to explain its logic. This not only makes it easier for developers to understand and collaborate, but it also helps prevent errors, and most importantly, it reduces the time and resources spent fixing them.
Following Coding Standards and Guidelines for Consistency
Coding standards establish consistent coding practices, including formatting, naming conventions, and commenting styles. Thanks to established standards, development teams can write cleaner, more maintainable code, avoiding these errors when programming. This leads to a more efficient development process and keeps everyone on the same page.
Collaborating with Peers for Code Reviews and Error Detection
Two pairs of eyes are better than one, which applies to coding. Code reviews offer a powerful weapon against errors because by having peers review each other's code, you leverage the combined knowledge of the development team.
Build a Strong Foundation for Your Next Project
Whether you plan to hire React developers or need broader development support, errors are a normal part of coding. Luckily, the steps described here can help your team identify and resolve issues efficiently. Grasping syntax, runtime, and logic errors will empower your business to maintain strong, stable foundations for your software.
Jalasoft's nearshore team augmentation services give you the access you need to skilled developers trained in a wide array of software development languages and best coding practices.
Contact us, and let us help you tackle errors when programming your next project.