C is often crowned as the fastest programming language. But is raw speed in a language from 1972 truly the holy grail for developers today? After all, it was the great Bjarne Stroustrup, the creator of C++, who said: "C makes it easy to shoot yourself in the foot".
This blog post dives deeper into this debate. We'll explore the top 10 fastest programming languages, analyze why speed might (or might not) be crucial for your project, and ultimately, help you decide if it's a primary factor when choosing a language.
And what exactly do we mean by "fastest"? It's not merely about how swiftly a language can produce lines of code. No, it's about execution speed, memory usage, and overall performance.What determines a programming language’s speed?
Several factors contribute to a programming language's speed:
Execution Efficiency
Optimization
Concurrency Support
Memory Management
Hardware Interaction
Just-In-Time (JIT) Compilation
Parallelism Support
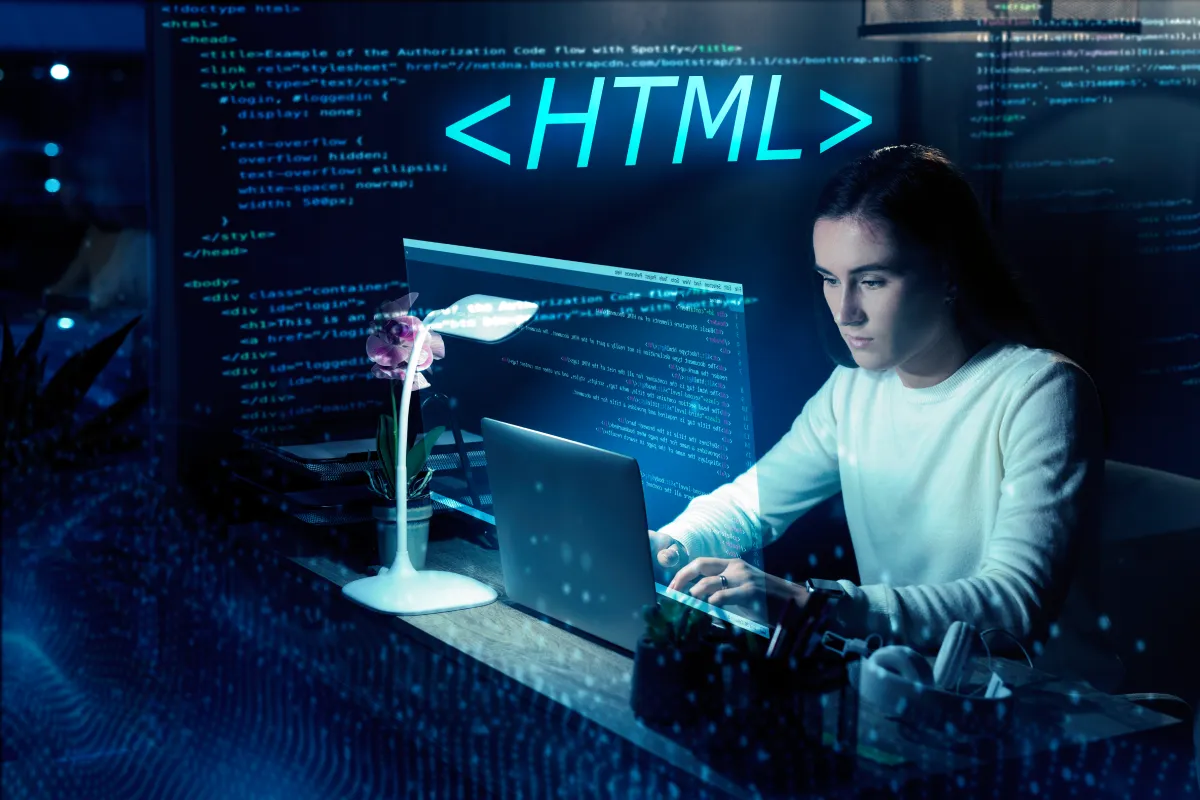
Compiled vs interpreted languages
Now that we've established that speed isn't the sole factor in choosing a programming language, let's delve into the two main categories that influence execution speed: compiled and interpreted languages. Compiled and interpreted languages vary in their processing and execution methods, which can significantly influence their speed and efficiency.
Compiled Languages:
Imagine a translator who meticulously converts an entire book (your code) into another language (machine code) that the computer can understand directly. Compiled languages work similarly. They translate your code into machine code before the program runs. This pre-translation eliminates the need for on-the-fly interpretation, resulting in generally faster execution speeds.
Compiled languages like C, C++, Go, and Rust prioritize raw speed. They achieve this by translating your code into machine code beforehand, using a compiler. This machine code acts as a pre-digested meal for the computer's processor, allowing it to execute the program much faster compared to on-the-fly interpretation. The result? Compiled languages often shine in performance-critical applications.
Interpreted Languages:
Think of an interpreter who reads the book (your code) line by line and translates it for the computer on the fly. Interpreted languages operate in this manner. Each line of code is interpreted and executed as the program runs. This approach offers more flexibility for development, but often comes at the cost of execution speed compared to compiled languages. Compiled languages are often preferred for performance-critical applications, whereas interpreted languages offer advantages in terms of portability and ease of development.
Interpreted languages, like Python, JavaScript, and Ruby, take a different approach. Instead of pre-compiling the entire program, they rely on an interpreter to translate the code line by line during runtime. Think of it like following a recipe step-by-step. This offers more flexibility for development but can sometimes lead to slower execution speeds compared to compiled languages.
However, it's important to remember that speed isn't everything. Interpreted languages often have other advantages, like faster development cycles due to simpler syntax and easier debugging. Additionally, some interpreted languages utilize techniques like bytecode compilation to achieve performance closer to compiled languages. In the next section, we'll explore the top 10 fastest programming languages, considering both compiled and interpreted options, to help you make an informed decision for your next project.
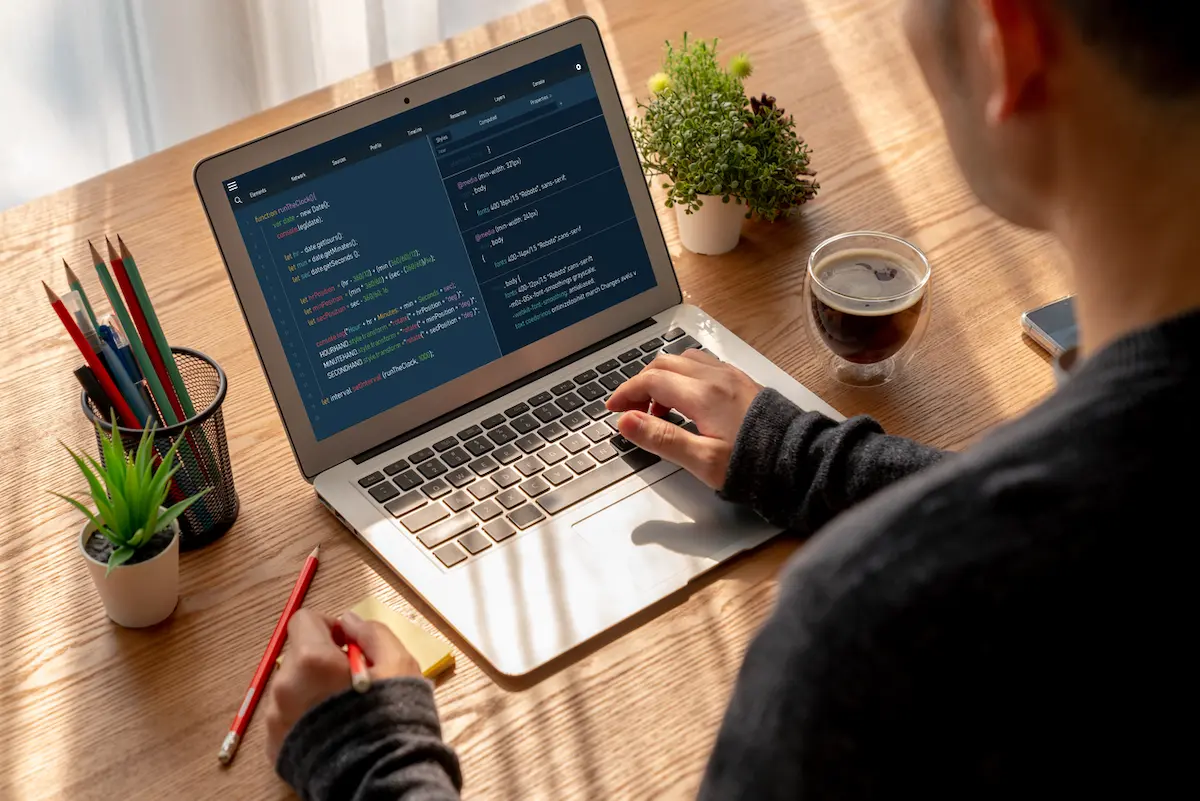
Top contenders for the fastest programming language
10. Pascal
In the early days of personal computers, Pascal emerged as a strong contender for the title of "fastest language." Developed by Niklaus Wirth in 1970, Pascal was known for its focus on efficiency and structured programming. Pascal reigned supreme as a language known for its speed. Compiled directly into machine code, Pascal offered significant performance advantages over its interpreted contemporaries like BASIC. However, several factors have contributed to Pascal's decline in the "fastest language" race:
Evolution of Compilers: Modern compilers for languages like C and C++ have become incredibly sophisticated, employing advanced optimization techniques like link-time optimization and auto-vectorization. These techniques squeeze every ounce of performance out of the code, often surpassing the raw speed achievable with Pascal's simpler compilation process.
Hardware Advancements: Modern processors are incredibly complex machines with features like out-of-order execution and deep pipelines. While Pascal was well-suited to the hardware of its time, it may not fully exploit the capabilities of modern CPUs, leaving some performance on the table.
Garbage Collection vs. Manual Memory Management: Pascal relies on manual memory management, which can be error-prone and requires careful handling to avoid memory leaks and performance degradation. Modern languages like C++ and Java, while not perfect, offer garbage collection that automates memory management, reducing development overhead and potential slowdowns.
Shifting Priorities: While raw speed was once paramount, development speed, code readability, and maintainability have become increasingly important factors in language choice. Pascal's focus on efficiency can sometimes come at the cost of code complexity, making it less attractive in today's development landscape.
Despite its fall from grace in the speed race, Pascal still holds value. Its clear syntax and emphasis on structured programming make it an excellent language for teaching programming fundamentals. However, for performance-critical applications, modern languages with advanced compilers, better hardware utilization, and more streamlined memory management have taken the lead.
9. F#
F#, a functional programming language developed by Microsoft, often enters the conversation about fast languages. Compiled to Common Language Runtime (CLR), F# offers some potential speed advantages. However, unlike some languages vying for the "fastest" title, F# prioritizes other aspects as well; like code clarity and readability, immutability, and expressiveness. Here's a breakdown of why F# might not be the absolute speed champion:
Focus on Functional Programming: F#'s core design principles prioritize code clarity, immutability (avoiding data modification), and expressiveness. While these features contribute to clean and maintainable code, they can sometimes lead to slightly less optimized machine code compared to languages like C++ that can leverage low-level control for maximum performance.
CLR Intermediary: F# code compiles to Common Language Infrastructure (CLI) bytecode, which is then interpreted by the CLR. While the CLR is highly optimized, this extra layer of interpretation can introduce a slight overhead compared to languages that compile directly to machine code.
However, F# shouldn't be completely discounted for performance:
Modern Compiler Optimizations: The F# compiler continues to evolve, incorporating advanced optimization techniques to improve code generation and execution speed.
Strength in Specific Domains: F# excels in areas like scientific computing and functional programming tasks. Its built-in features and libraries can streamline development and potentially achieve competitive performance in these domains.
Ultimately, F# shines for developers who value a balance between performance, code readability, and functional programming paradigms. While it might not be the absolute top contender in the raw speed race, F# can deliver efficient execution for various applications, particularly when its functional strengths align well with the project's requirements.
8. C#
C#, another Microsoft-developed language, sits firmly in the realm of high-performance contenders. Compiled to Common Language Runtime (CLR) bytecode, C# offers a good balance between speed and other desirable qualities. Here's a look at why C# might not be the absolute speed champion:
CLR Intermediary: Similar to F#, C# code goes through an extra layer of interpretation by the CLR before execution. This can introduce a slight overhead compared to languages that compile directly to machine code.
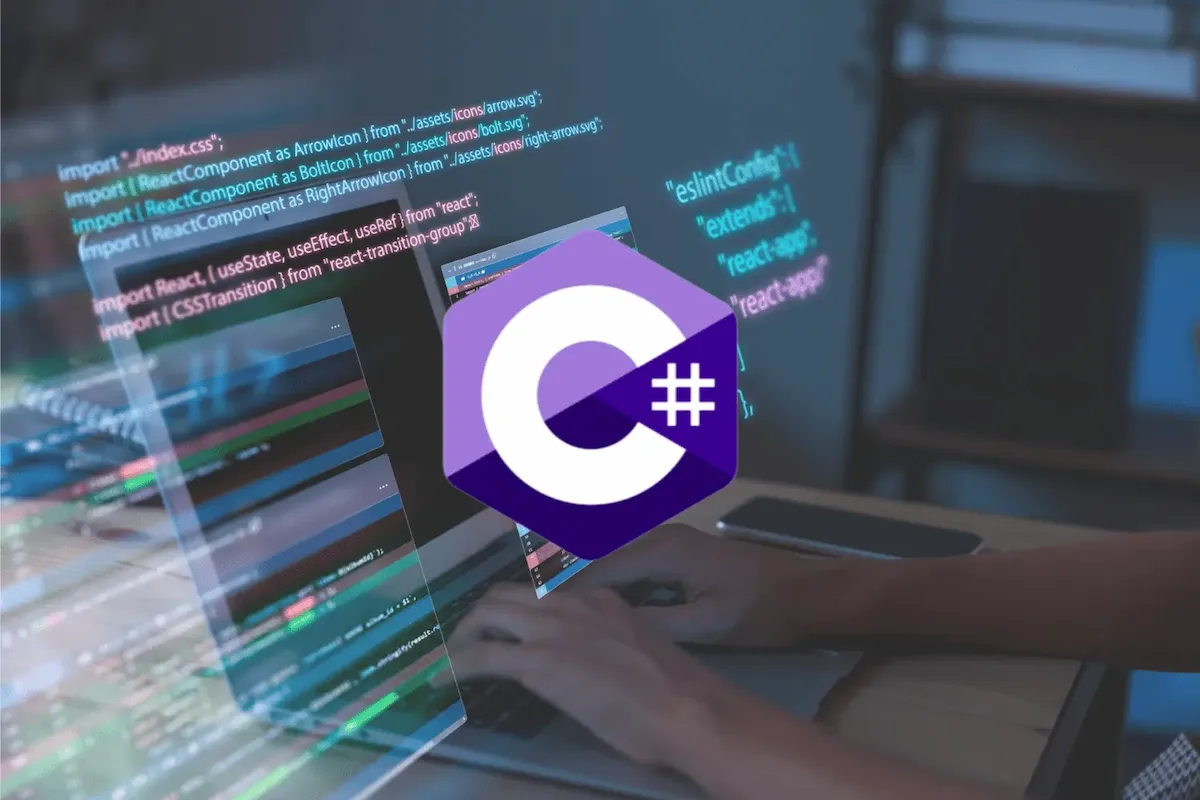
However, C# boasts several features that contribute to its overall performance:
Modern Compiler Optimizations: The .NET compiler platform (Roslyn) is constantly evolving, incorporating advanced optimization techniques to generate efficient machine code from C# source code.
Just-In-Time (JIT) Compilation: The CLR employs Just-In-Time (JIT) compilation, where frequently used code sections are compiled to native machine code at runtime. This allows for further performance gains as the application executes.
Large and Mature Ecosystem: The .NET ecosystem surrounding C# offers a wealth of libraries and frameworks that are often highly optimized for performance. Developers can leverage these tools to build performant applications without reinventing the wheel.
C# strikes a balance between raw speed, developer experience, and a vast ecosystem of tools and libraries. This makes it a popular choice for a wide range of applications, from game development to enterprise software. While it might not be the absolute leader in the pure speed category, its overall performance capabilities, combined with other strengths, make it a compelling option for many projects.
7. Java
Despite being an interpreted language, Java deserves a mention for its ability to achieve good performance through techniques like Just-In-Time (JIT) compilation. The Java Virtual Machine (JVM) can compile frequently used code sections to native code at runtime, leading to significant performance improvements. Here's what makes Java interesting:
Platform Independence: Java code can run on any platform with a JVM, making it a versatile choice for applications that need to be portable across different operating systems.
Large Ecosystem and Developer Base: Java boasts a vast ecosystem of libraries and frameworks, along with a large community of developers. This makes it easier to find resources and support for Java development projects.
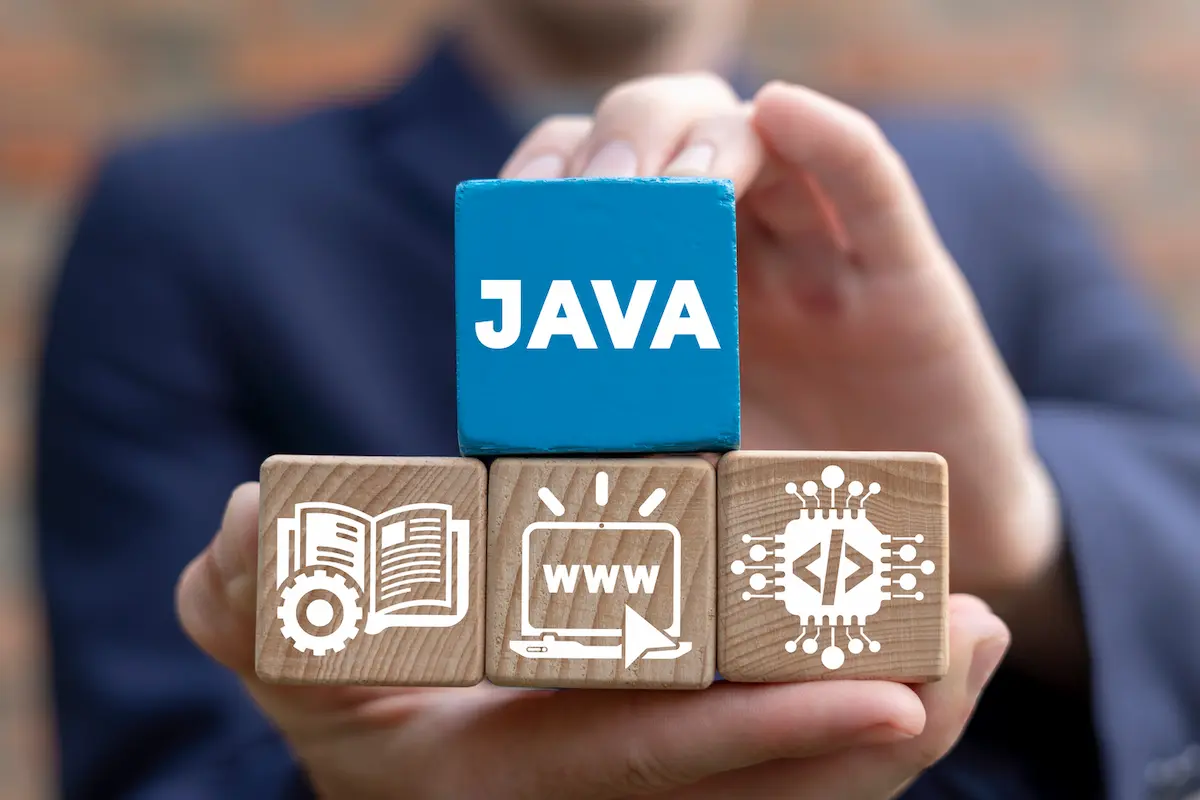
However, limitations exist:
Startup Time Overhead: Interpreted languages like Java typically have a slower startup time compared to compiled languages. This might not be a major concern for long-running applications, but it can be noticeable for programs that launch frequently.
Not Always the Fastest Choice: While JIT compilation helps, Java may not always match the raw speed of compiled languages like C++ for highly performance-critical tasks.
6. Ada
Ada, designed with safety and reliability in mind, offers good performance alongside robust features for error handling and real-time programming. While not the absolute fastest language, Ada excels in applications where safety and predictability are crucial. Examples include:
Avionics: The critical nature of flight control systems necessitates reliable and predictable performance, which Ada's features can help ensure.
Air Traffic Control Systems: Similar to avionics, air traffic control systems require reliable operation to prevent accidents. Ada's focus on safety makes it a suitable choice for these applications.
Military Software: Military systems often have strict requirements for reliability and real-time performance, which Ada can deliver.
However, some trade-offs exist:
Potential Performance Overhead: Ada's safety features can sometimes introduce a slight overhead compared to languages like C. This might not be a significant concern for safety-critical applications, but it's a factor to consider.
Less Widely Used: Compared to more mainstream languages, Ada has a smaller community and a less extensive ecosystem of libraries and frameworks.
5. Julia
Julia combines high performance with a user-friendly syntax, making it accessible to scientists who may not be programming experts. This allows them to focus on the scientific problem rather than wrestling with complex language constructs. Here's what makes Julia appealing:
Expressive Syntax: Julia borrows elements from familiar languages like Python and MATLAB, offering a readable and concise way to write code. This reduces the learning curve for scientists who are comfortable with these existing tools.
Performance on Par with Established Languages: Benchmarks show Julia performing competitively with Fortran and C in many scientific computing tasks. This allows scientists to achieve high-performance results without sacrificing code readability or maintainability.
Growing Ecosystem: The Julia community is actively developing new libraries and frameworks specifically tailored for scientific computing needs. This expanding ecosystem offers powerful tools for various scientific domains.
However, some considerations exist:
Relative Immaturity: Compared to Fortran's long history, Julia is still under development. This means the language and its ecosystem might be less stable and have fewer resources compared to more established options.
Limited Adoption Outside Scientific Computing: While Julia shows promise, its focus on scientific tasks means it might not be as widely used for general-purpose programming compared to other languages on this list.
4. Fortran
For decades, Fortran has been a dominant force in scientific computing, and its focus on speed remains a major strength. Originally designed for numerical calculations, Fortran compilers are highly optimized for scientific operations. While not as versatile as some newer languages, Fortran excels in specific domains like:
Scientific Simulations: Fortran's optimized libraries and mature ecosystem make it a powerful tool for modeling complex physical phenomena.
Weather Forecasting: Weather prediction models often rely on Fortran's efficiency for processing vast amounts of data and performing complex calculations.
Computational Fluid Dynamics: Simulating fluid flow and other engineering problems benefits from Fortran's ability to handle large datasets and perform calculations quickly.
However, some limitations exist:
Limited Application Outside Scientific Computing: Fortran's syntax and standard library are geared towards scientific tasks, making it less suitable for general-purpose programming.
Modernization Efforts Ongoing: While efforts are underway to modernize Fortran with features like object-oriented programming, it may not be as user-friendly as some newer languages.
3. Rust Rust has garnered significant attention in recent years for its focus on both speed and memory safety. Unlike C and C++, where manual memory management is crucial for achieving high performance, Rust employs a unique ownership system. This system eliminates the need for manual memory management, preventing memory leaks and crashes that can plague performance-critical applications. Rust's combination of these features makes it a compelling option for:
Systems Programming: Rust's memory safety and performance make it suitable for developing reliable operating system components, embedded systems, and device drivers.
High-Performance Applications: Rust's speed allows it to excel in areas like web servers, network applications, and real-time systems.
Concurrent Programming: Rust's ownership system simplifies concurrency by ensuring memory safety when dealing with multiple threads.
However, keep in mind:
Steeper Learning Curve: Like C++, Rust's ownership system introduces a new paradigm that can take time to master, especially for developers coming from languages with garbage collection.
Evolving Ecosystem: While Rust's ecosystem is rapidly growing, it might not yet have the vast amount of libraries and frameworks available compared to more established languages like C++.
2. C++
C++ builds upon C's foundation, offering a balance between raw speed and programmer control. It introduces object-oriented features like classes and inheritance for better code organization and memory management compared to C. However, C++ still allows access to hardware for high performance. This combination makes C++ a popular choice for:
Game Development: Modern game engines rely heavily on C++ for rendering graphics, physics simulations, and achieving smooth gameplay performance.
Performance-Critical Applications: C++ is used in various domains like financial trading systems, scientific computing libraries, and high-performance servers.
System Programming: C++'s ability to interact directly with hardware makes it suitable for developing device drivers and low-level system components.
1. C C reigns supreme in the realm of raw speed. Compiled directly to machine code, C grants meticulous control over hardware interactions. Programmers can extract every ounce of performance from the system, making C ideal for applications where speed is paramount. These include:
Embedded Systems Programming: C's efficiency is crucial for resource-constrained devices with limited memory and processing power.
Operating System Development: The core components of operating systems often rely on C for their performance-critical tasks.
High-Performance Computing: Scientific simulations and other computationally intensive tasks leverage C's speed to achieve faster results.
However, C's power comes with a price:
Complexity Due to Low-Level Nature: C requires manual memory management, which can be error-prone and lead to memory leaks if not handled meticulously. This complexity increases development time compared to higher-level languages.
Steeper Learning Curve: C's syntax and concepts can be challenging for beginners to grasp, requiring a deeper understanding of computer architecture and memory management.
Final Verdict: Which is the fastest programming language?
Our exploration has shown that C reigns supreme in the raw speed arena. Compiled directly to machine code, it grants meticulous control, allowing programmers to squeeze every ounce of performance from the system. However, the power of C comes with a double-edged sword. While C offers the ultimate speed potential, achieving it heavily relies on the programmer's skill and the available libraries or frameworks. A well-written program in a higher-level language like Java or C# can often outperform poorly written C code, especially when considering factors like development time and maintenance. Additionally, extensive libraries and frameworks in these languages can provide optimized functions and algorithms, negating some of the raw speed advantages of C.
C: Speed with Potential Peril
C's fundamental strength – its low-level nature – also becomes its Achilles' heel. The ability to directly manipulate memory and hardware interactions is a double-edged sword. In the hands of an experienced developer, it leads to exceptional performance. However, for those less familiar with its intricacies, C's lack of safeguards like automatic memory management and strong type checking can lead to:
Security vulnerabilities: Memory leaks and buffer overflows can create security holes that attackers can exploit.
Program crashes: Dangling pointers and other memory-related errors can cause unpredictable crashes and instability.
Increased development time: Debugging and fixing errors in C can be time-consuming due to the language's low-level nature.
Perhaps the most crucial point to consider is that most languages beyond C aren't actively chasing the title of "fastest language." They prioritize developer experience, code maintainability, and a rich ecosystem of libraries. These languages are deliberately willing to sacrifice some raw performance in exchange for increased developer productivity and safer, more secure code. This trade-off allows developers to build complex applications more efficiently, often achieving sufficient performance for most use cases.
Ultimately, the best language choice depends on your project's specific needs. When raw speed is paramount, and you have highly skilled developers who can handle C's complexities, it might be the ideal option. However, for most projects, the benefits of developer productivity, code readability, maintainability, and security offered by higher-level languages often outweigh the slight performance gains achievable with C. Consider all factors – the skill set of your team, the complexity of the project, and the desired balance between performance and development efficiency – when making your decision.
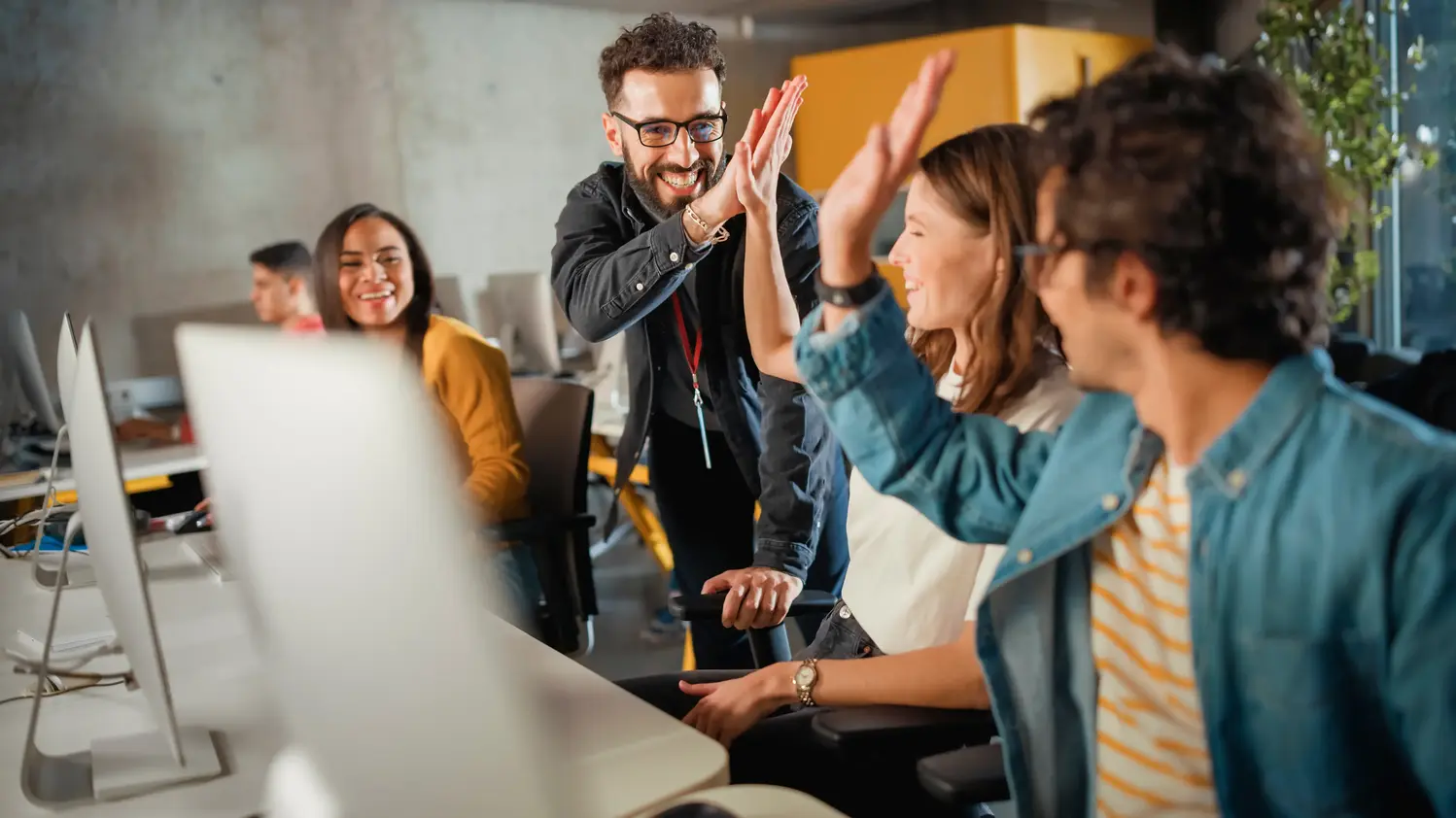
(Discover the benefits of an extended development team model and how it can boost your software projects)
Jalasoft: Your Partner in Choosing the Right Language
At Jalasoft, we understand the complexities of choosing the right programming language for your project. Our team of nearshore software development experts possesses a vast knowledge of various languages and technologies. We can help you analyze your project requirements, assess your team's skill set, and recommend the language that best aligns with your needs for performance, development efficiency, and overall project success. Contact us today to discuss your project and explore how Jalasoft can empower you to build exceptional software solutions.