Did you know 47% of users expect a webpage to load in 2 seconds or less? Slow loading times can lead to frustrated visitors and, consequently, lost revenue. Companies like Netflix and PayPal have seen significant improvements in performance, including a reduction in page loading times by up to 60%, and all thanks to the implementation of Node.js.
But this is just one of the advantages of using Node.js. This open source server environment optimizes web development, helping businesses build high-performance applications. With Node.js, developers can use a single programming language (JavaScript) for both the frontend and backend, which accelerates development times, reduces costs, and delivers a greater user experience. All critical elements for success.
So, whether you're building a new website or revamping an existing one, Node.js can offer your business a powerful solution.
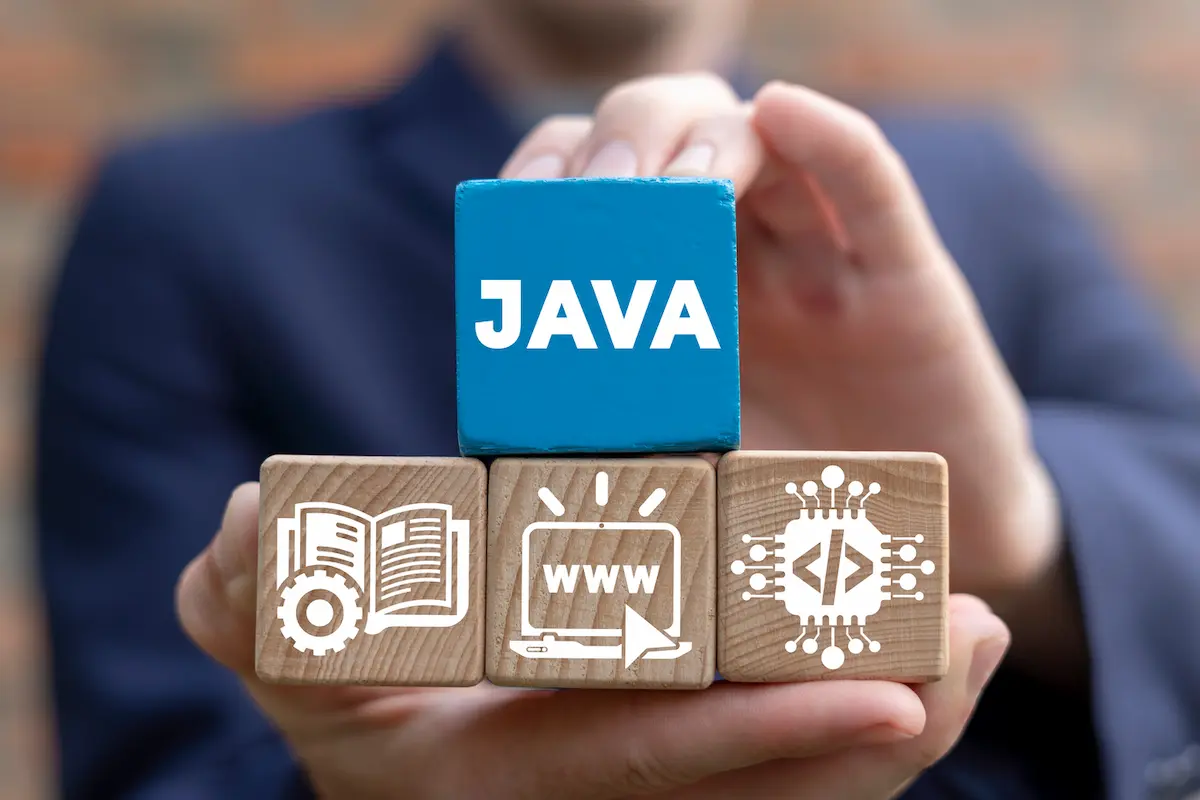
Understanding Node.js
Overview of Node.js
Node.js is an open-source JavaScript runtime that facilitates the creation of scalable network applications. Launched in 2009 by Ryan Dahl, it supports both frontend and backend development using a single language, which naturally simplifies development.
As Node.js offers a way to run JavaScript outside the browser, it boosts overall project efficiency, whether running an express application or managing development tasks with a package manager.
Key Features of Node.js
Node.js is renowned for its non-blocking I/O model, which improves both efficiency and scalability by handling multiple tasks at the same time. A vast package manager (npm) and its active community make it possible to build high-performing and scalable applications.
Advantages of Using Node.js
Lightning-fast performance thanks to Google's V8 engine.
Fast, non-blocking architecture for efficient handling of user requests.
Manage multiple tasks simultaneously for a responsive user experience.
Use one language (JavaScript) for both server-side and client-side development.
Rich package ecosystem (npm) for easy access to pre-built functionalities.
Common Use Cases for Node.js
Node.js adapts to a variety of development needs. It's ideal for building express applications and complex single-page apps (SPAs) while it is very effective for creating chat and gaming applications where real-time updates are crucial. With its powerful package manager, Node.js simplifies code management, improving the development of data streaming and command-line applications.
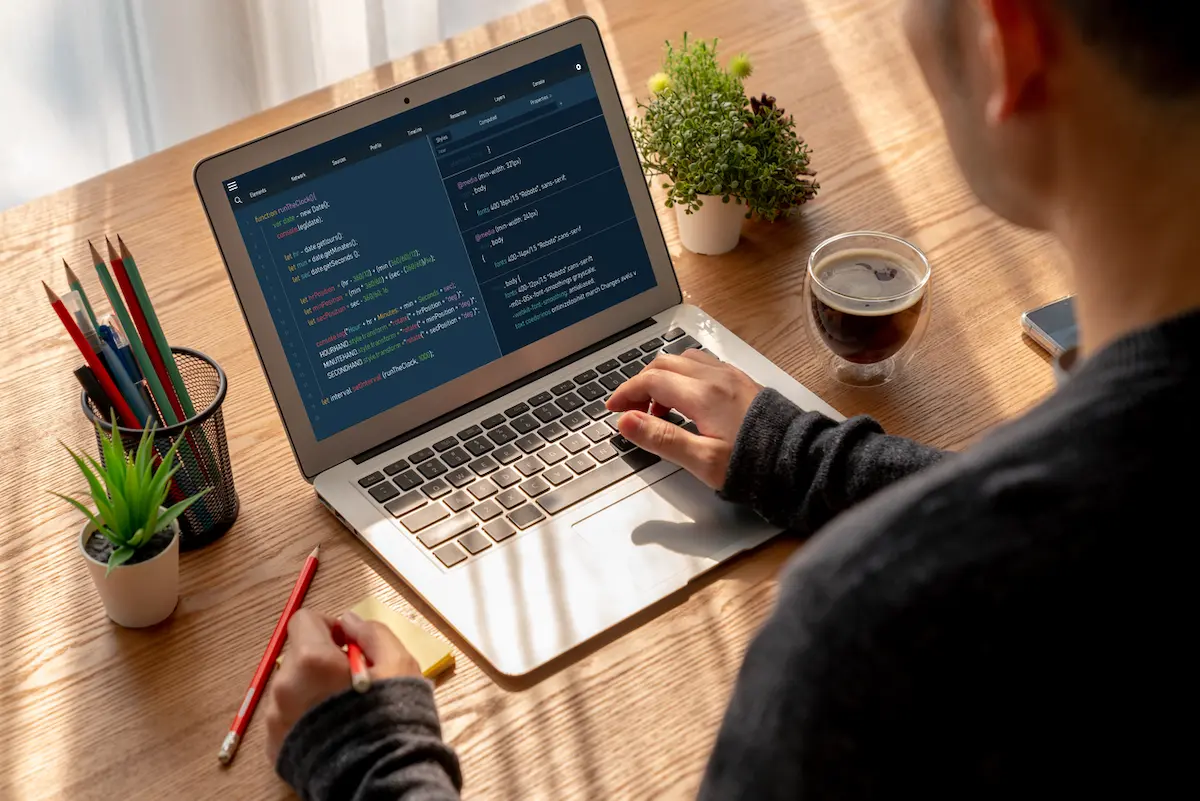
Building Your First Node.js Application
1. Creating a Basic Node.js Server
To create a basic Node.js server, start by installing Node.js and npm. Next, create a project folder and navigate to it in your terminal. Run npm init -y
to generate a package.json
file. Install Express with npm install express
, then write your server code in a file like app.js
. And finally, run your Node.js application using node app.js
to start the server and begin the development.
2. Integrating Express.js Framework
This popular framework simplifies server setup and lets you write clean and organized code. To integrate Express.js into your Node.js application, start by installing the Express package using npm. Simply run npm i express
to add it to your project dependencies and get access to its features. Now, you can build your express application with a solid foundation for handling server requests and routing.
3. Implementing Middleware for Request Processing
Middleware functions are custom-built to handle tasks like authentication, logging, and error management, and it's executed in a specific sequence, with each function able to pass control to the next using next()
. To implement middleware for request processing, start by installing Express using your package manager. You can use Express to integrate these functions into your Node.js app by calling app.use()
to run your middleware code.
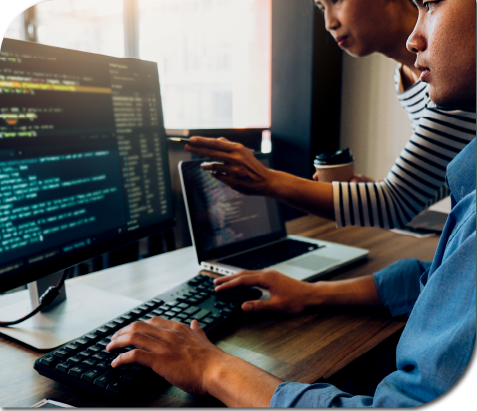
Working with Data and Databases
Connecting Node.js to Databases
Node.js works flawlessly with several databases. To connect your Node.js application to a database, start by installing the appropriate package for your database. For MySQL, use the mysql2
module; for PostgreSQL, the pg
module; and for MongoDB, the mongodb
module. Once installed, integrate these packages into your Node.js code to run queries and handle data effectively.
Performing CRUD Operations with MongoDB
Using MongoDB with Node.js, you can easily insert, query, modify, and remove documents. To work with MongoDB in an application, you'll use methods to create, read, update, and delete (CRUD) documents. To integrate these with your Node.js application, you can use MongoDB's methods, such as db.collection.insertOne()
to add documents and db.collection.find()
to retrieve them.
Utilizing Mongoose for Object Data Modeling
When working with data in Node.js, Mongoose simplifies working with MongoDB databases by providing a schema-based data modeling system. This Object-Data Modeling (ODM) library helps you define and manage your data structure efficiently. Consequently, this approach makes sure that your application’s development is both scalable and maintainable, supporting multiple types of data-intensive projects.
Testing and Debugging in Node.js
1. Writing Unit Tests with Mocha and Chai
Testing and debugging are critically important in Node.js development. Mocha, a flexible JavaScript test framework, makes writing and maintaining tests straightforward with its clean syntax and support for asynchronous testing. Paired with Chai, an assertion library, developers can easily verify that their express application code delivers the expected results. This catches issues early and keeps your Node.js project on track!
2. Debugging Node.js Applications
Use debuggers to walk through the code line-by-line, inspect variables, and identify issues. To start debugging an application in Node.js, insert a debugger
statement in your code. Then, run node inspect yourfile.js
to launch debug mode. Avoid over-relying on console.log();
instead, take advantage of advanced debugging tools like Visual Studio Code or Node.js Inspector. These tools are invaluable for debugging synchronous and asynchronous code, helping streamline the software QA process and ensuring a more robust application.
3. Using Postman for API Testing
API testing is essential for ensuring your web services perform as planned, and Postman simplifies this process by allowing you to build, test, and manage APIs with a user-friendly interface. Download the Postman app, set up your environment, and use it to create, run, and automate tests for your APIs. This will ensure your code is reliable and your Express application meets all functional requirements.
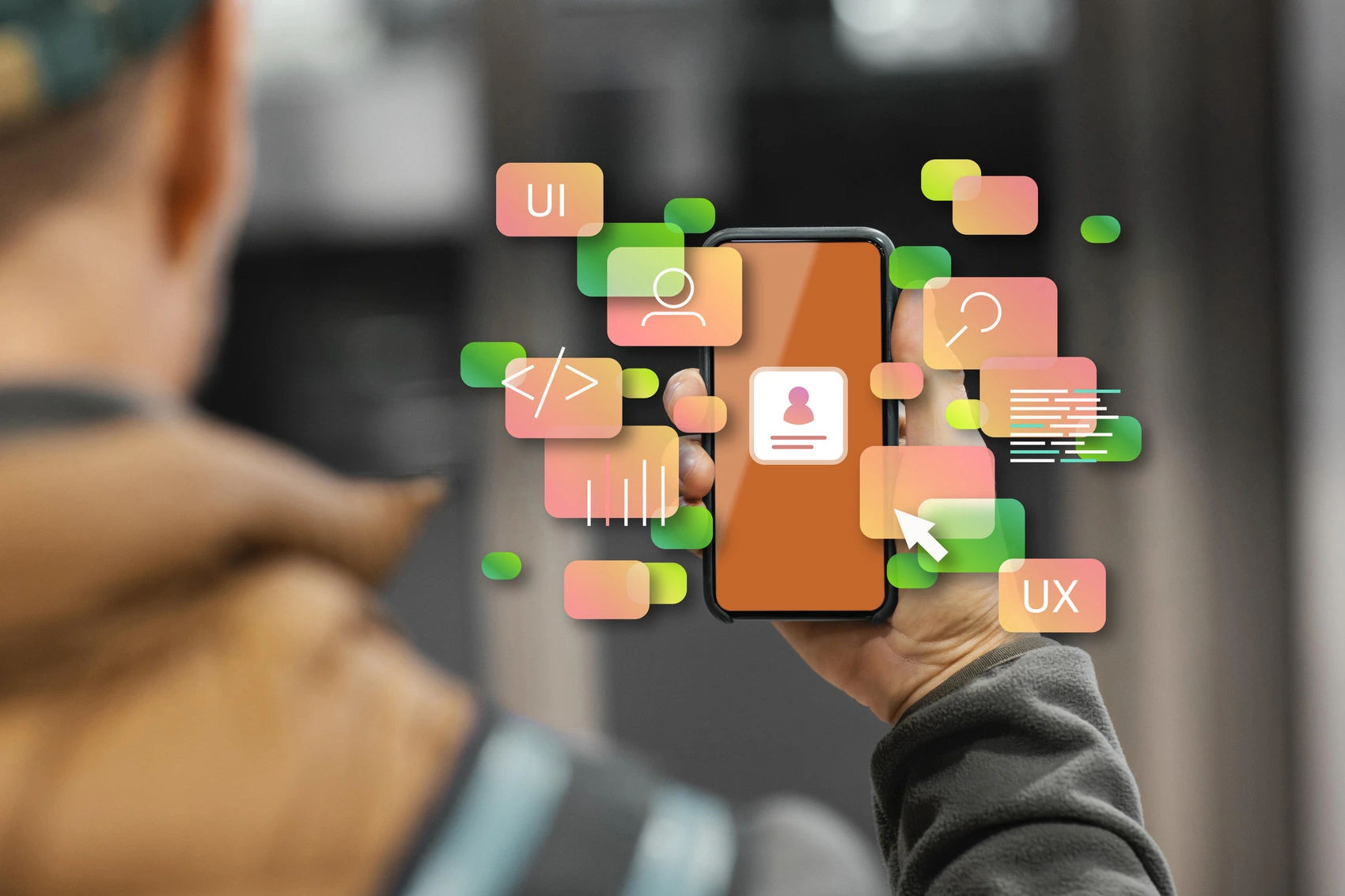
Deploying Node.js Applications
Choosing a Hosting Provider
Opt for a provider that supports the event-driven nature of Node.js and offers reliable, secure services. Evaluate traffic expectations, support, and flexibility. You can choose between managing your own cloud VM or opting for a managed cloud hosting platform. These managed platforms simplify deployment and maintenance, letting you focus exclusively on the code.
Setting Up Continuous Integration/Continuous Deployment (CI/CD)
Deploying applications efficiently requires setting up Continuous Integration and Continuous Deployment (CI/CD). Begin by using a version control system like Git, then select a CI/CD tool such as GitHub Actions. Define workflows in a YAML file, integrate automated testing, and use tools like Docker for deployment. You can also consider containerization for smoother deployment across environments.
Monitoring and Scaling Node.js Applications
To ensure your application scales effectively, you can implement a microservices' architecture to manage individual components independently, and use containerization tools like Docker. Additionally, employ monitoring tools to track performance, and configure auto-scaling to adjust resources based on demand. These practices will help maintain a consistent execution and reliability as the Node.js app grows.
Advanced Concepts in Node.js
1. Understanding Asynchronous Programming in Node.js
Asynchronous programming in Node.js allows tasks to run independently, making the application more responsive. Node.js takes advantage of this approach through mechanisms like callbacks, promises, and async/await. For example, in an Express application, these techniques enable non-blocking operations, ensuring the app handles multiple requests efficiently without delay.
2. Exploring WebSocket Communication with Socket.io
WebSocket communication transforms how servers and clients interact by establishing a continuous, bidirectional channel, enabling two-way communication between your Node.js server and browsers. Unlike standard HTTP, servers can push updates to clients, creating a more dynamic user experience. Therefore, with Node.js and Socket.IO, you can integrate WebSocket into your application, boosting interactivity and reducing server load.
3. Implementing Real-Time Applications with WebSockets
Real-time applications take advantage of WebSockets in Node.js to provide an instant data exchange between clients and servers, allowing you to push real-time updates to clients. This means that, by integrating WebSockets into your Node.js app, you will be improving user experiences with features like live updates and instant messaging, all while using frameworks like Express for a more efficient development.
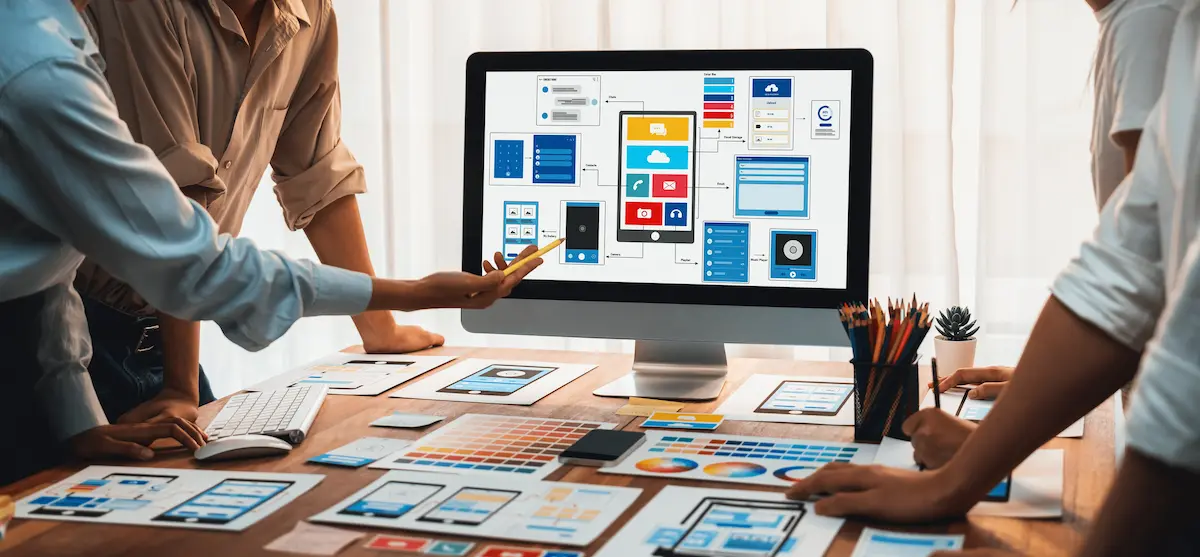
Next Steps to Develop Successful Node.js Applications
Mastered the fundamentals of Node.js? It's time to build exceptional applications! As you explore the features of Node.js and its role in developing high-performing apps, the next step is to maximize its full potential. Whether you're building an express application or scaling an existing Node.js project, our staff augmentation services can equip you with the expertise needed for success.
Contact us to assess how Node.js fits into your development strategy and ensure you have the right tools and talent for success.