React is a free, open-source JavaScript library. It’s widely used for building modern web applications; maintained by Meta and a community of its users, it enables developers to create dynamic, efficient, and responsive user interfaces (UI). It’s mainly known for its simplicity: you can use individual pieces, called components, and combine them into screens, pages, and apps.
React is known for its ability to manage Document Object Model (DOM) updates. It achieves this by only updating pages in response to effective changes in its components. In short, unnecessary rerendering is avoided, which is a very practical measure as it reduces the performance cost.
However, despite React's optimized process, it’s not perfect—hardly anything is. There are times when developers may need to force a rerender. Understanding how and when to do it—and how and when to do it well—is the subject of today’s article.
Buckle up because we’re going to cover a lot of ground. First, we’re going to delve into the concept of rerendering; then, we’re going to discuss why it’s important; finally, we’re going to suggest some common good practices. We will also explore strategies for optimizing performance: `shouldComponentUpdate`, `PureComponent`, and the `key` prop.
What Is Rerendering in React?
“Rerendering” refers to the process where React updates the user interface (UI) in response to changes in the component's state or props. When a component’s state or props change, React “rerenders,” calculating its output and updating the corresponding parts of the DOM. This ensures that the UI stays in sync with the application’s underlying state. It’s a very practical feature, as it ensures clarity in the process of building the UI.
React uses a tool called virtual DOM (VDOM) to optimize the rendering process. When a component is about to rerender, React first updates the VDOM. Then, in a process known as “reconciliation,” React compares the new virtual DOM with the previous version. This determines the most efficient way to update the actual DOM. Only the differences between the old and new virtual DOM are applied to the real DOM —thus optimizing the performance and avoiding unnecessary re-rendering.
Types of Rerenders
There are two main types of rerender. The first and more traditional one is called “initial rerender” and occurs when a component is mounted for the first time. The second is called “subsequent rerender” and is triggered when a component’s state or props change. In that case, React rerenders the VDOM to reflect the updated data in the UI.
However, sometimes, these two types of automatic rerender are not enough. Automation only takes you to a certain point. Manual intervention may be necessary, in some cases, to optimize or control when a certain component rerenders.
Why Is Rerendering Important in React?
Rerendering is crucial because it keeps the user interface consistent with the underlying data. Without rerendering, any changes to the application wouldn’t be reflected in the UI, which can lead to a broken or inconsistent user experience. Efficient rerendering is especially important for applications with dynamic content, such as social media feeds, real-time data updates, or interactive forms.
However, in the last few years, applications have grown in size and complexity. Today, UIs are easier to use but harder to develop. In those cases, excessive rerendering can negatively impact performance: rendering too many components can cause slowdowns, particularly in large-scale applications with many nested components. That’s why many developers want to be careful with the way they force or optimize rerenders in React.
Significance of Forcing Rerender
React typically manages rerenders automatically, but as we’ve already discussed, there are scenarios where you may need to manually force it. This can be useful, for example, when you are dealing with very complex user interfaces: you may want to improve the performance by forcing a rerender only in strategic points.
There are also cases when React doesn’t detect a change. In some instances, an external library or a non-React component modifies its state, but React doesn’t notice it, so it doesn’t trigger the rerender automatically. This can happen, too, if you mutate the state directly without creating a new object or array.
That being said, forcing rerenders, as with many other actions, should be done cautiously. No remedy is good when used in excess. Overuse of forced rerenders can lead to performance issues, and they can bypass React’s efficient reconciliation process.
When Does React Rerender?
React triggers a rerender of a component in many scenarios. Most of them are automatic, therefore, linked to changes, and there’s also one—the one we’re most interested in today—which is manually triggered.
The first of these circumstances we’ve already talked about is when the component’s state changes. Updating the state with the setState() method causes React to rerender the component, as well as its children (if needed, of course). Similarly, if the component receives new props, React will rerender it to reflect them. The same happens when the component subscribes to a context and the context value changes. This ensures that any changes in the context are reflected throughout the component tree.
The last case—the case we’re mainly dealing with in this article—is when the forceUpdate() method is explicitly called. This forces React to rerender the component, regardless of whether the state or props have changed. It’s useful for certain advanced scenarios (that we’ll soon discuss in detail).
By default, React only triggers a rerender when it detects a change in state or props. However, there is a caveat: it does so only if the new values are different from the previous ones. If you erase something but then replace it with the exact same value, it will not re-render. This avoids unnecessary work and improves the overall performance.
Common Issues That Require Force Rerender
As we’ve promised before, we can now discuss some common scenarios where a developer may need to force a rerender.
One frequent issue arises from what’s called a direct state mutation. It goes like this: to trigger rerenders, React relies on detecting state changes. But what happens if the state is mutated directly, such as modifying an object or array in place? React won’t notice it and, in consequence, the component won't rerender. We’re going to talk more about this in the next section, but in the meantime, we can advance a solution: calling `forceUpdate()`.
Another common situation involves external libraries or APIs. Sometimes, they modify component states outside of React’s management system—they might directly update the DOM, for example. React won’t automatically detect that. Here, forcing a rerender with `forceUpdate()` can help synchronize the UI with the changes.
There are other changes that can go undetected by React, such as the ones involving nested data structures or arrays. They are complex and therefore, they can be tricky. If React doesn’t detect them, you might need to trigger a rerender to reflect the new state manually.
Lastly, for now, we have to talk about complex interactive UIs. Certain user actions may need to trigger UI updates at specific moments. In these cases, calling `forceUpdate()` can maintain the synchronicity.
Implementing Force Rerender in React
But you came here to find methods for triggering a rerender. We’ve arrived. Now, we’re going to discuss three of them, probably the most used: `setState()`, `key` prop, and `forceUpdate()`. These three tools should be enough to manage most of the problems with rerendering in React.
Using the `setState` Method
Out of the three tools we're going to discuss in this article, the `setState()` is perhaps the most common one. It causes React to update the component's state, which automatically triggers a rerender. Here you can see it in action:
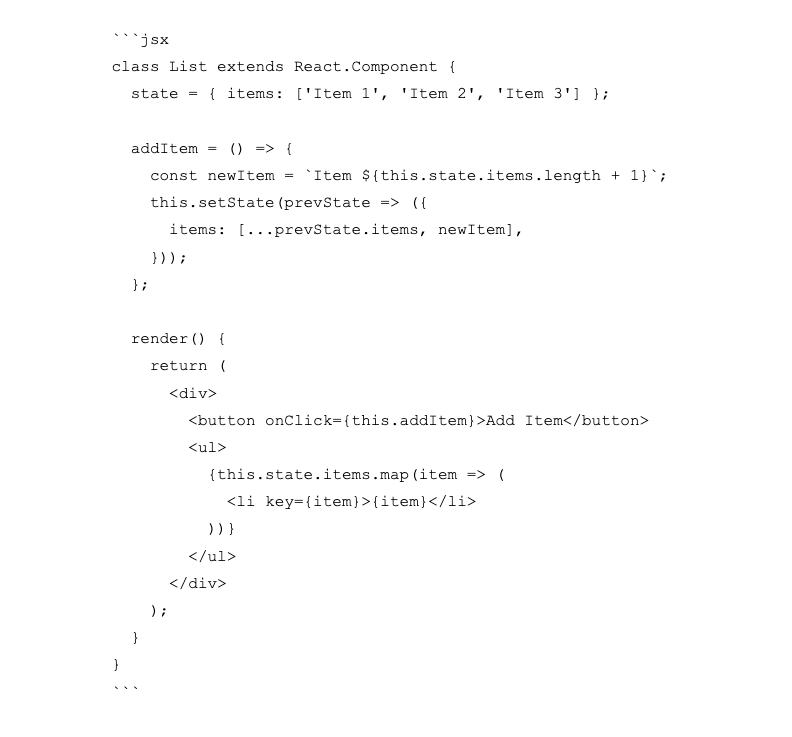
In this case, each time the button is clicked, the `handleIncrement` function updates the state, triggering a re-render.
Manipulating the `key` Prop
The `key` prop is used to identify elements in a list. Then, React optimizes updates by triggering them when the list
In this example, when the `key` prop for each list item changes, React forces a rerender, ensuring the list updates correctly.
Utilizing the `forceUpdate` Method
Last but not least, we have `forceUpdate()`. This method is typically used as a last resort, when the other two failed or were not useful. In those instances, you can use `forceUpdate()` to trigger a rerender regardless of anything else. Here’s an example:
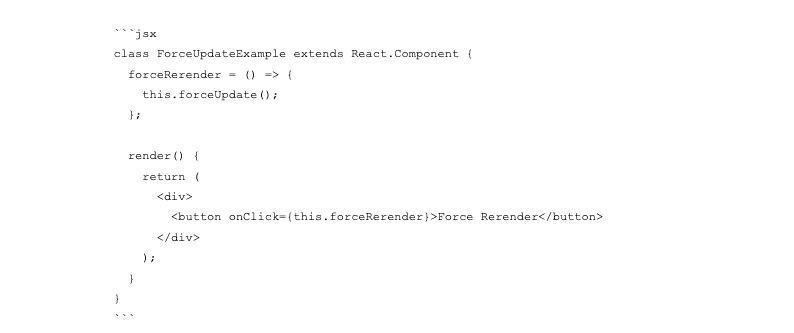
`forceUpdate()` is useful, but it should be used in moderation. Bypassing React’s normal state and props-checking mechanism can lead to other problems, like diminishing the performance.
Enhancing Performance with Rerender Optimization
As we discussed, forcing rerenders can sometimes be necessary, but frequent forced rerenders can negatively impact performance. There’s an equilibrium to be found. That’s why, to avoid most problems, React provides several optimization strategies that can help you minimize unnecessary rerenders.
Implementing `shouldComponentUpdate` and `PureComponent`
React provides a very useful tool to enhance performance: the `shouldComponentUpdate` lifecycle method.
As you know, by default, React rerenders a component whenever its state or props change; however, this tool allows you to control the moment at which a component should rerender, preventing unnecessary rerenders.
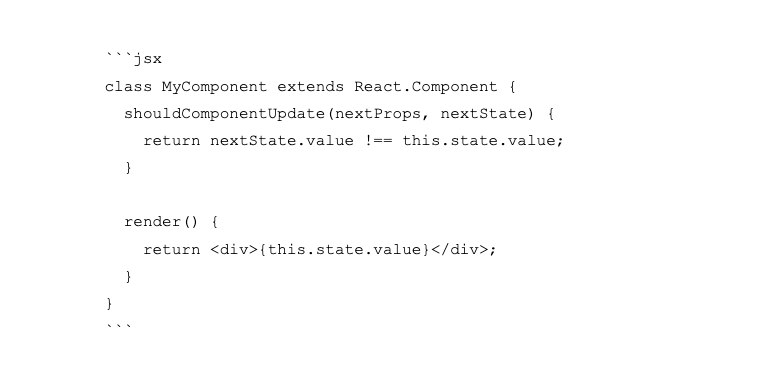
`PureComponent` is a similar but simpler tool. What does it do? `PureComponent` automatically implements `shouldComponentUpdate` (with a shallow comparison of props and state).
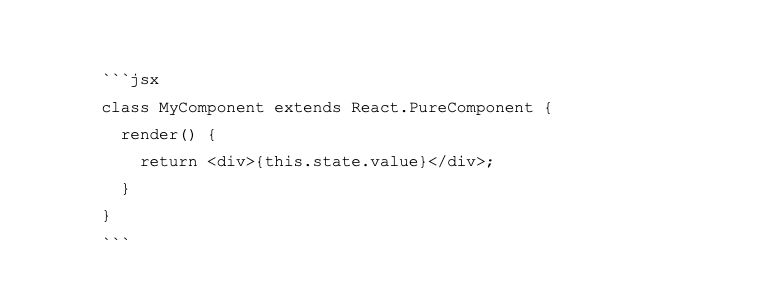
Avoiding Unnecessary State Updates
Any developer knows you can’t take common sense for granted. So, here’s a somewhat obvious warning: to avoid unnecessary rerenders, ensure that you only update the state when necessary. In other words, if the state hasn't changed, avoid calling `setState()`.
Utilizing `key` Prop for List Item Optimization
The `key` prop deals with lists. It has a critical function: it allows React to quickly identify which items have changed, been added, or been removed. Logically, as rerendering is automatically linked to changes, this results in a more efficient process.
When to Avoid Force Rerendering
As valuable as knowing when to rerender is knowing when not to do it. The most obvious situation in which you should avoid a rerendering is when React can handle the update itself: if React is already triggering rerenders based on state or prop changes, there's no need to force an update. Additionally, force rerenders should be avoided when performance is critical; they can bypass React’s optimizations, especially in large applications. Excessive rerenders can slow UI interactions and longer load times—and no user wants that.
Maximizing Efficiency with React Rerender
Maximizing efficiency should be one of every development team’s top priorities. Accomplishing it in React requires, first, optimizing updates and then ensuring your components are updated in a performance-friendly way. As we’ve discussed in the previous section, `shouldComponentUpdate`, `PureComponent`, and the `key` prop are the developer’s main allies in this fight.
Conclusion
We’ve discussed the main issues surrounding rerendering: what is it, the difference between forced and automatic rerenders, when to trigger them (and when not to), and how. It’s a vital tool. React’s automatic process is highly efficient and usually doesn’t need much help, but that doesn’t mean it’s perfect. There are still scenarios where human intervention is necessary. Knowing these situations, understanding their mechanisms, and implementing best practices can save time and effort and, more importantly, can help developers in their work of creating high-performance applications with smooth and responsive user experiences.