Are you ready to dive into the world of building dynamic web applications from the ground up using a single language? Get ready to streamline your development process and create exceptional user experiences.
What is Node.js?
Node.js is an open-source, cross-platform runtime environment that allows developers to execute JavaScript code on the server side. Built on the V8 JavaScript engine used in Google Chrome, Node.js provides a fast and efficient way to build scalable, high-performance applications.
Key Features of Node.js
The power of Node.js relies on its fast, interesting features that allow high-quality products. Let’s revise some of them:
Asynchronous and Event-Driven: Node.js handles multiple concurrent connections efficiently without creating new threads for each request. This non-blocking architecture makes it ideal for I/O-bound applications.
Single-Threaded: While it's single-threaded, Node.js leverages the event loop to handle multiple requests concurrently.
High Performance: Built on Chrome's V8 JavaScript engine, Node.js offers impressive speed and efficiency.
Scalability: Its ability to handle multiple concurrent connections makes it highly scalable for applications with growing user bases.
Large Ecosystem: A vast array of open-source modules and packages available through npm (Node Package Manager) accelerates development.
Full-Stack Development: Developers can use JavaScript for front-end and back-end development, streamlining the development process.
In essence, Node.js empowers developers to create fast, scalable, and efficient applications with JavaScript.
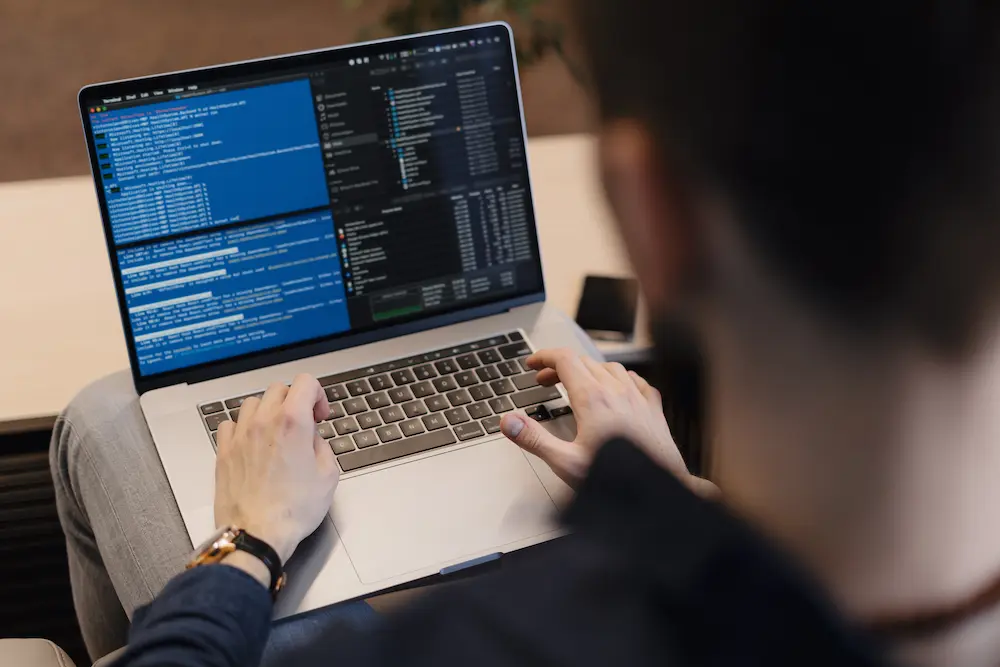
Node.js vs. Traditional Server-side Technologies
Comparison with PHP, Java, and C#
Node.js has disrupted traditional server-side development with its asynchronous, event-driven approach, challenging established technologies like PHP, Java, and C#.
While PHP excels in content-heavy websites and smaller projects, and Java dominates enterprise applications, Node.js shines in real-time, high-traffic environments.
C# offers versatility across web, enterprise, and gaming. The optimal choice depends on project needs, considering scalability, performance, and team expertise.
Ultimately, the best technology—Node.js, PHP, Java, or C#—is determined by specific project requirements and long-term goals.
How Node.js Works
Event-Driven Architecture
At the heart of Node.js is the event loop, a single-threaded mechanism that continuously processes events and enables non-blocking I/O operations. This allows Node.js to handle tasks asynchronously, without waiting for I/O operations like reading files or querying databases to complete.
Key features include callbacks, promises, and async/await for managing asynchronous tasks, and the EventEmitter class for creating and handling custom events.
This architecture is well-suited for real-time applications, API servers, and microservices, offering exceptional scalability, performance, and responsiveness.
Node.js's event-driven model ensures high concurrency and efficient resource utilization, making it ideal for applications requiring low latency and high throughput.
Asynchronous Programming in Node.js
Node.js thrives on asynchronous programming. This approach allows multiple tasks to run concurrently without halting the application's progress.
The event loop, Node.js's core mechanism, manages these asynchronous operations efficiently. Non-blocking I/O operations, such as file reading or network requests, are essential for maximizing performance.
While callbacks were initially used, the potential for complex code structures led to the development of Promises and async/await for more manageable asynchronous workflows.
By embracing these techniques, developers can create highly responsive and scalable Node.js applications.
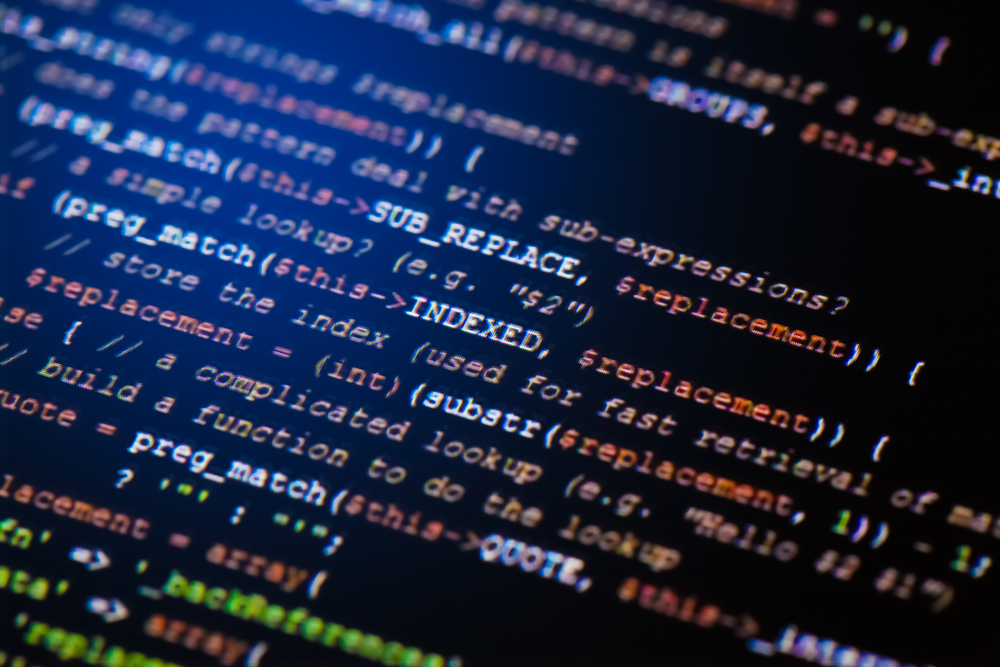
Getting Started with Node.js
Installation of Node.js
A straightforward way to install Node.js is through a package manager, with each operating system having its package manager. Additional package managers for macOS, Linux, and Windows can be found at Node.js Package Managers.
Another popular method is using nvm (Node Version Manager), which facilitates switching between different Node.js versions, installing new ones, and rolling back if necessary. This tool is handy for testing code across various Node.js versions. More details about nvm can be found at nvm GitHub.
Once Node.js is installed, you'll have access to the node executable in your command line, allowing you to start building and running Node.js applications.
Basic Node.js Commands
Once you have Node.js installed, you can start building and running applications easily. Here are some essential Node.js commands that will help you get started and manage your projects effectively.
node
: Executes a JavaScript file.node -e "console.log('Hello, world!')"
: Runs a single-line JavaScript code snippet.node -v
: Displays the installed Node.js version.npm -v
: Displays the installed npm (Node Package Manager) version.
For more complex scripts, consider using a shebang line at the beginning of your JavaScript file: #!/usr/bin/env node. This allows direct execution without the node command.
Node Package Manager (NPM)
Introduction to NPM
Node Package Manager, commonly known as npm, is a crucial tool for any Node.js developer. It is the world's largest software registry, containing over 1.3 million packages that provide reusable code to simplify and streamline your development process.
npm is a command-line tool that facilitates the installation, updating, and management of third-party packages and modules in Node.js projects. It comes bundled with Node.js, meaning that when you install Node.js, npm is automatically installed as well.
Some of its key features include package management, registry, version control, scripts, and publishing.
In essence, npm is a powerful tool that simplifies development by providing access to a vast ecosystem of reusable code.
Managing Packages with NPM
On the other hand, managing packages with npm is vital for Node.js developers, simplifying dependency handling.
Devs can install packages locally with npm install package-name or globally with npm install -g package-name.
Also, uninstall using npm uninstall package-name (local) or npm uninstall -g package-name (global).
Moreover, update packages with npm update package-name or npm update for all.
Check for outdated packages using npm outdated, or add development dependencies with npm install package-name --save-dev and list installed packages with npm list (local) or npm list -g (global).
Lastly, ensure security with npm audit and npm audit fix. And, run scripts defined in package.json with npm run script-name.
Mastering these commands helps manage dependencies efficiently and maintain a secure, up-to-date codebase.
Creating a Simple Node.js Application
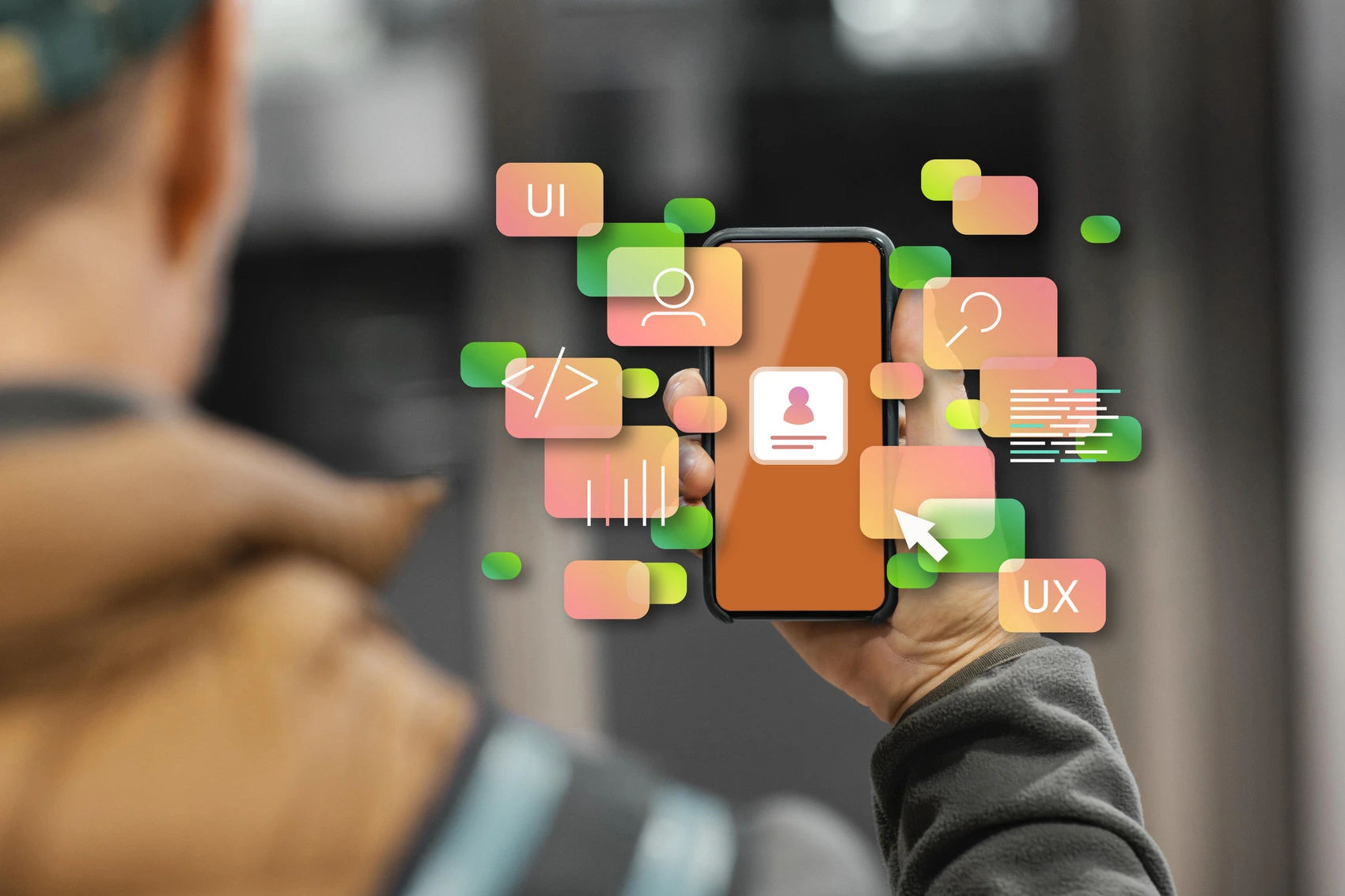
Setting up a Basic Server
Creating a basic Node.js server involves initializing a project with npm, installing the express framework, creating a JavaScript file to define routes and server configuration, and finally starting the server. This foundational setup allows you to build upon it by adding more routes, handling different HTTP methods, and integrating other functionalities.
Handling Requests and Responses
Handling requests and responses in Node.js is key for creating functional web servers and using the Express framework simplifies this process.
First, install Express with npm install express. Set up a basic server in a server.js file, then use app.get() for handling GET requests and app.post() for handling POST requests.
Middleware such as express.json() is used to parse incoming JSON data. Define routes like app.get('/', (req, res) => { res.send('Hello, World!'); })
and app.post('/data', (req, res) => { console.log(req.body); res.send('Data received'); })
to manage different types of requests and send appropriate responses.
This setup ensures efficient handling of client-server interactions in your Node.js application.
Node.js Best Practices
Optimizing Node.js Performance
Optimizing Node.js performance is crucial for delivering fast and responsive applications. Key strategies include asynchronous programming, caching, profiling, clustering, code optimization, and database optimization.
The combination of these techniques can significantly enhance your Node.js application's speed and efficiency.
Security Considerations in Node.js Development
Node.js security is crucial for protecting applications and user data. Key areas include input validation to prevent attacks like SQL injection and XSS, robust authentication and authorization, secure session management, and error handling to avoid exposing sensitive information.
Encrypting data with HTTPS, regularly updating dependencies, and conducting security audits are essential steps.
Prioritizing security will allow developers to build trustworthy Node.js applications!
Node.js Use Cases
Real-world Applications of Node.js
Real-world applications use Node.js. It powers web applications like content management systems (CMS) and e-commerce websites for companies like Ghost, eBay, and Walmart. Real-time applications such as chat apps and online gaming platforms benefit from Node.js's ability to handle immediate data updates and interactions.
Node.js is also popular for building RESTful APIs and microservices, with companies relying on it for scalable API services.
Additionally, Node.js is ideal for IoT applications, managing multiple concurrent connections for smart home devices and other connected technologies.
Benefits of Using Node.js in Web Development
This technology has numerous advantages for web development. Its asynchronous, non-blocking architecture excels in building real-time applications, I/O-intensive tasks, and high-performance servers.
Using JavaScript for both frontend and backend development streamlines development and fosters code reuse. Additionally, Node.js boasts a vast, active community and a rich ecosystem of packages, accelerating development and problem-solving.
All in all, Node.js empowers developers to create innovative and scalable web applications.
(Node or Python? Which one should you choose for your next project? Discover Which Technology is Better for Back-end Development)
Node.js Community and Resources
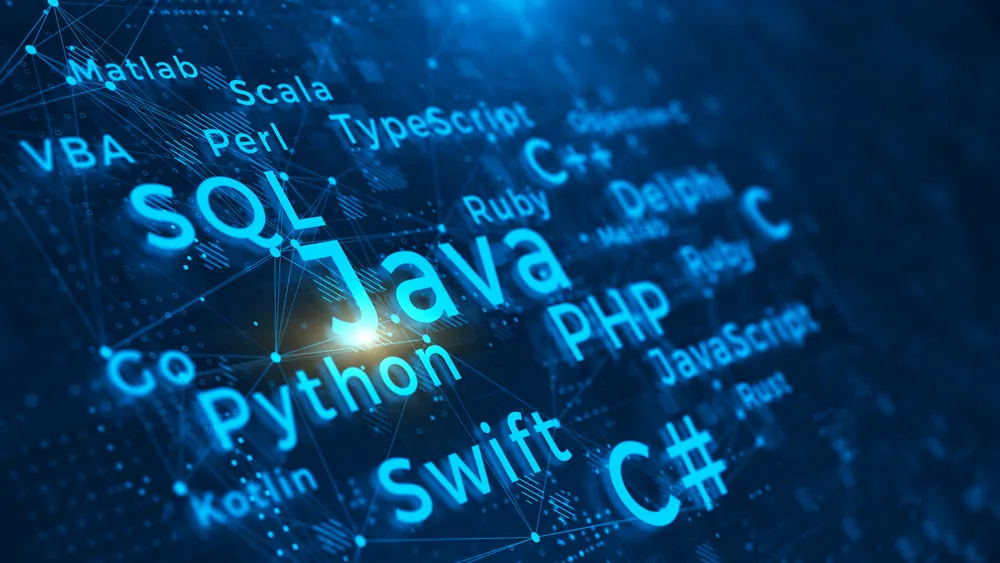
Engaging with the Node.js Community
The Node.js community is a vibrant hub for developers, offering invaluable resources and opportunities to learn, contribute, and grow. Actively engage with the community to stay updated on the latest trends, best practices, and potential job opportunities. Remember, the Node.js community is a powerful network for both personal and professional growth!
Recommended Learning Resources for Node.js
Did you know that you can master Node.js by combining official tutorials, interactive platforms like NodeSchool, and practical projects? Enhance learning with books and online courses. Engage with the community through forums, meetups, and open-source contributions. Consistent practice is key.
Future Trends in Node.js Development
Emerging Technologies Impacting Node.js
It is no mystery that emerging technologies are reshaping Node.js development.
AI and machine learning integration, serverless architectures, and GraphQL adoption are gaining traction. Real-time applications, microservices, and IoT continue to be strong use cases. As Node.js evolves, developers must stay updated on these trends to build cutting-edge applications
Predictions for the Future of Node.js
The future of Node.js looks bright, with increased adoption in enterprise solutions due to its scalability and performance.
We can expect enhanced performance and security through ongoing improvements to the V8 engine and runtime. The growth of serverless architecture will lead to more Node.js applications on platforms like AWS Lambda and Azure Functions, simplifying deployment and scalability.
Additionally, the Node.js ecosystem will continue to expand with new libraries, frameworks, and tools, further enhancing its capabilities and developer productivity.
Overall, Node.js is poised to remain a leading platform for modern web development!
Conclusión
Mastery of Node.js is the first step towards building exceptional applications. To truly maximize its power, you need expert guidance. Our team at Jalasoft specializes in harnessing Node.js for high-performance projects. From real time app development to scaling existing Node.js solutions, we provide the expertise you need.
Let's discuss how Node.js can drive your project forward. Contact us today!