React is a powerful and widely used JavaScript library that simplifies the creation of interactive user interfaces for web applications. It utilizes building blocks called “components.” These components act like mini-applications, each responsible for a specific aspect of the user interface.
But how do these components come to life, update, and eventually disappear? This guide explores the concept of the component lifecycle, highlighting the different phases (mounting, updating, and unmounting) and the methods called at each stage.
We'll explain how components interact with props and state, how updates trigger re-renders, and the role that constructor, render, and componentDidUpdate methods, among others, play in this process.
However, while understanding the so-called components can be valuable, building and managing complex React applications often requires the expertise of highly skilled developers. By partnering with our trained professionals at Jalasoft, you can ensure the development process leverages React's full potential to build high-performing and scalable applications.
By the end of this guide, you'll gain valuable insights into the complexities of the React component lifecycle and appreciate the expertise required to develop a successful project.
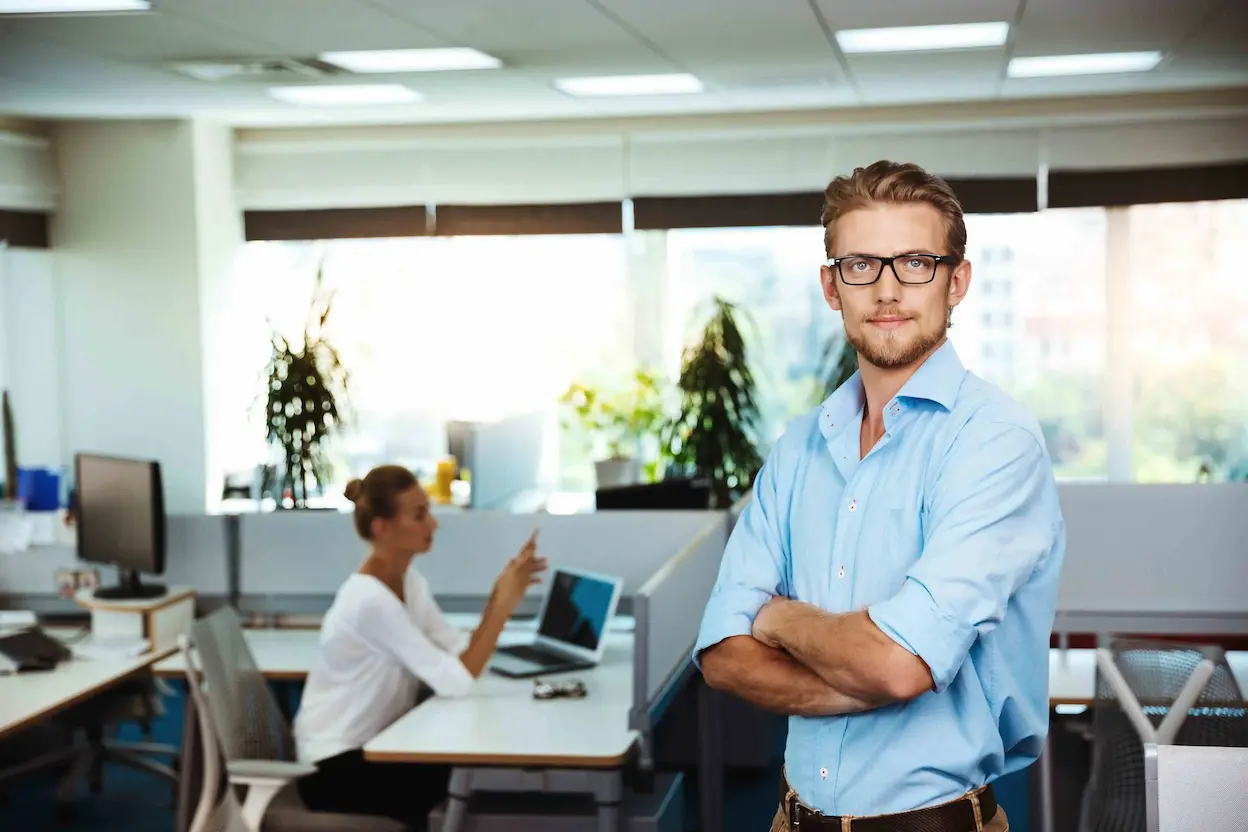
What is a Component in React?
React's adoption rate is impressive. Over 15% of the top 1 million most trafficked websites use React to power their user interfaces, translating to roughly 31,591,018 million websites globally.
Interfaces like e-commerce platforms and social media feeds are often built using modular parts called components.
React components follow a specific lifecycle. This lifecycle consists of several phases: mounting, updating, and unmounting. During these phases, various lifecycle methods allow developers to control the component’s behavior at different stages. For example, during the mounting phase, the constructor method is called to initialize the component. Following this, the render method displays the component on the screen.
Overall, the power of React is rooted in its ability to combine these components efficiently. Components can be nested within other components, creating a hierarchical structure that mirrors the complexity of the application.
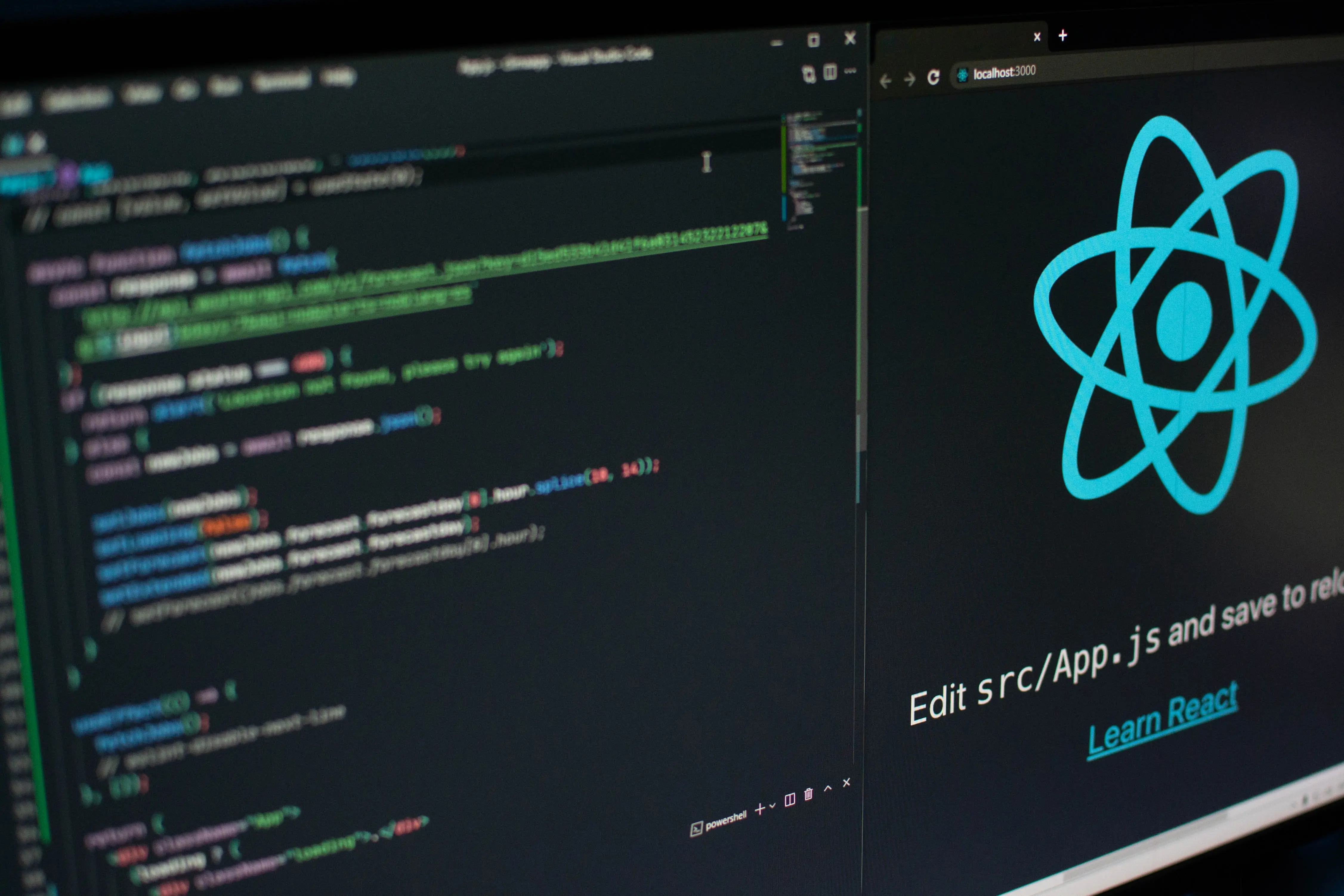
The React Component Lifecycle
Understanding the React component lifecycle is essential for optimizing development projects. This lifecycle is a series of methods called at different stages of a component's existence. And here’s when the three main phases behind the scenes of the React component lifecycle become protagonists.
Mounting phase: This is when a component is first introduced into the application. Here, the component is initialized, any initial data or state is set, and the core building block, the render method, is called for the first time. The render method takes the component's state and props (external instructions) and determines how the component should appear on the screen. Once rendered, the component is inserted into the Document Object Model (DOM).
Updating phase: This phase kicks in whenever a component's state (or props) changes. React detects these changes and triggers a re-render of the component. The component re-evaluates its state and props, updates its internal data if necessary, and then calls the render method again.
This is how these key methods in this phase work:
-The static getDerivedStateFromProps, updates the state based on prop changes before the render method is called again.
- After the render method, the getSnapshotBeforeUpdate method captures information before the DOM is updated, and the componentDidUpdate method is called to operate on the updated DOM.
- The shouldComponentUpdate method determines if a re-render is necessary.Unmounting phase: The final stage occurs when a component is no longer needed and is removed from the DOM. This usually happens when a user navigates to a different page or section within the application. The component cleanup methods like componentWillUnmount are called at this stage, allowing developers to perform any necessary tasks to optimize performance.
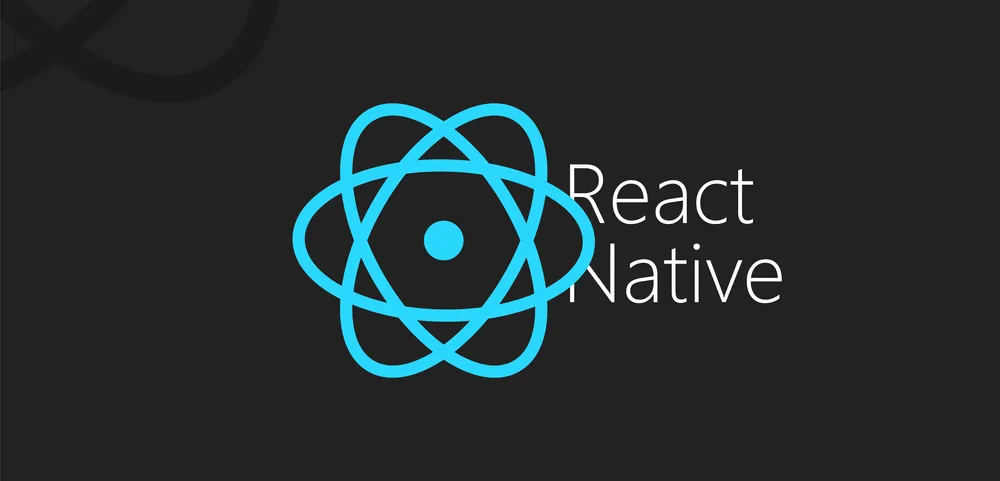
React Hooks
While the core lifecycle methods provide a solid foundation for building components, the introduction of "React Hooks" in version 16.8 opened doors to even more flexibility and code reusability. Traditionally, managing a component's internal state required using the lifecycle methods and the constructor to set up the initial state, while class components relied on the componentDidUpdate method and getSnapshotBeforeUpdate method to manage props and state.
Hooks allow the use of state and other features within functional components, which are generally considered simpler and easier to reason about.
Here's an example:
Imagine a component displaying a counter that increases with each click. Without Hooks, developers would define the component as a class, manage the counter value as a state, and utilize lifecycle methods to update the state and re-render the component on each click.
Hooks, on the other hand, provide a function called useState that allows developers to declare and manage state within a functional component directly. However, certain actions within a component, like fetching data from an API, might be considered "side effects" as they occur outside the typical render process.
To manage these side effects, the useEffect hook replaces lifecycle methods such as the componentDidMount, componentDidUpdate, and componentWillUnmount methods. This hook allows a function to run after the render method, making it ideal for tasks like data fetching or manually modifying the DOM. The ability to specify dependencies ensures that the effect is only called when necessary, improving performance.
The useContext hook enables sharing values like global state or props across a component tree, streamlining the data flow, especially in complex applications where multiple child components need access to the same data.
To sum up, while the concept of hooks might seem technical, their impact is clear: cleaner, more maintainable, and ultimately, more efficient React applications.
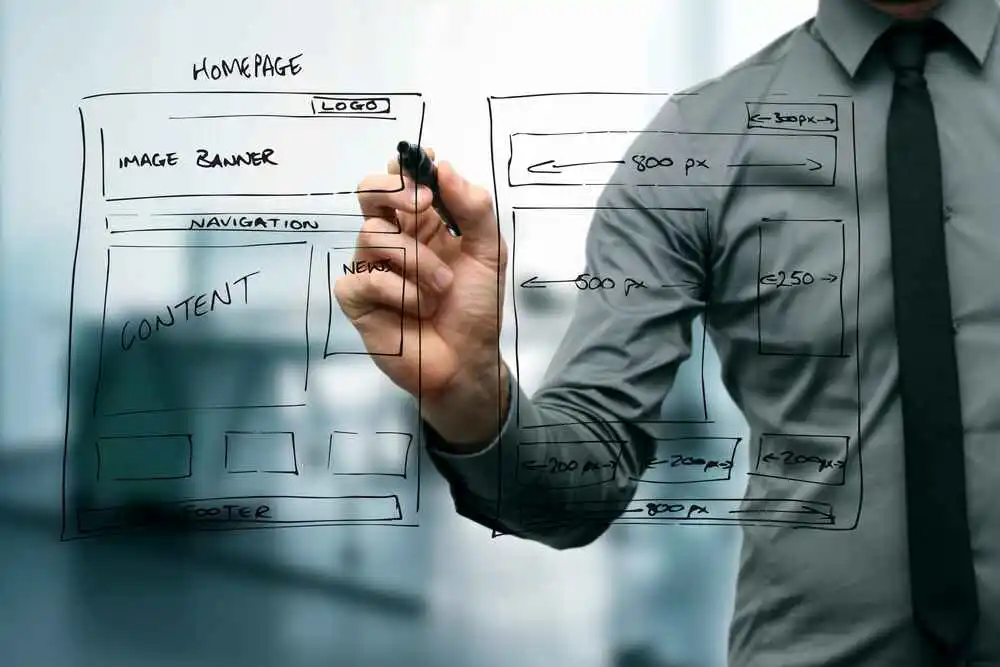
React Apps
Today's most popular web and mobile applications use React to deliver exceptional user experiences. From a news feed that updates in real-time using the render method to a social media platform where content loads and refreshes with a method called component, up to an e-commerce website where product pages adjust to user interactions and preferences using props state, these are all examples of the power of React.
Major applications like Facebook, Instagram, Netflix, and WhatsApp exemplify the effectiveness of React. In fact, Facebook utilizes ReactJS for its web page and React Native for its mobile app, leveraging the method called component and the update method to ensure smooth user experiences.
Here's how React can benefit businesses:
Enhanced user experience: React is particularly good at creating interactive and responsive user interfaces through the effective use of props state and the render method.
Scalability and performance: As your business grows, so too can the application. The modular nature of the library’s components allows for easier scaling and maintenance, ensuring your application remains updated even with increasing user traffic. By using a method called component, developers can easily add new features without disrupting the existing structure.
Faster development cycles: The focus on reusability and clear code structure speeds up the development process. This allows for a faster time-to-market for a web application, providing a significant competitive advantage.
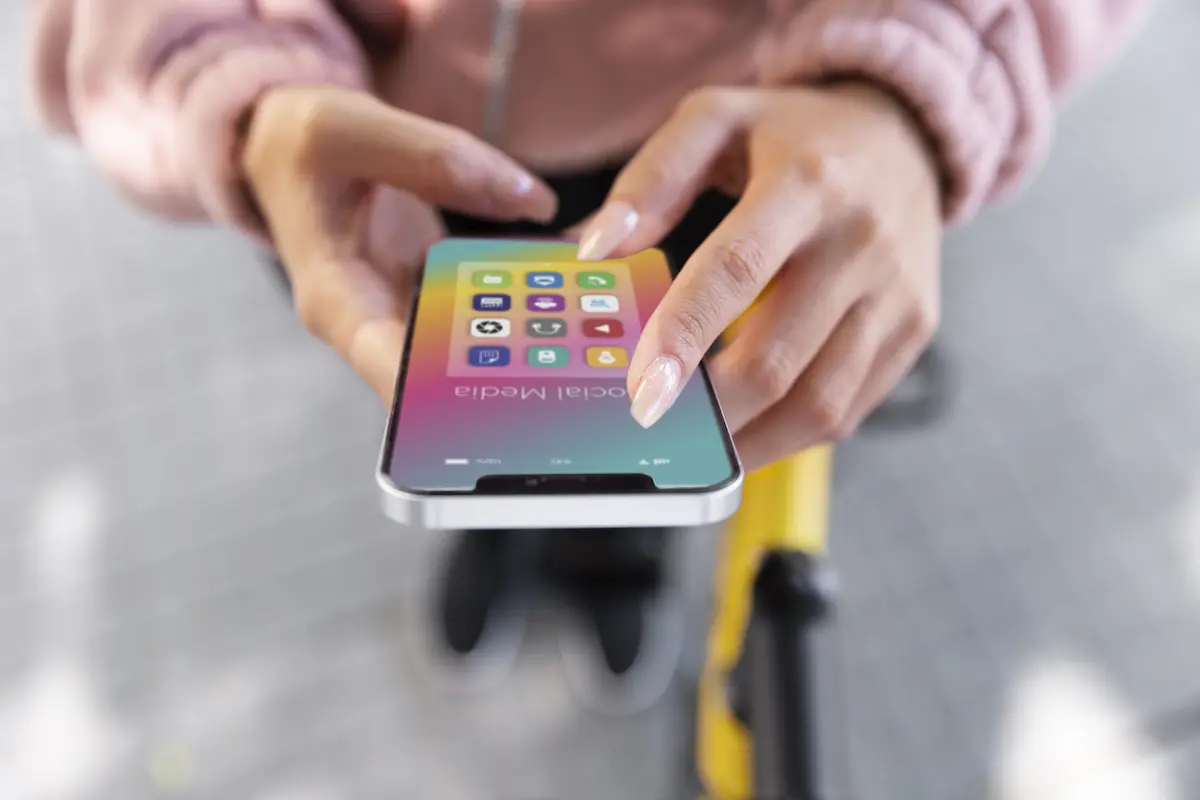
Bringing a React App to Life: Connection and Deployment
Once the application is developed and complete with well-defined component lifecycles, it's time to connect it with the real world. This process involves two steps: connection and deployment.
Connection refers to establishing a link between the app and the data it interacts with. Imagine a product catalog app, the connection would bridge the gap between the components and the product database, allowing users to see real-time information.
During this phase, methods such as the constructor and the method called during the mounting phase are crucial. These methods set up the initial props state and ensure the app is ready to fetch and display data efficiently.
Deployment is the process of making the app go live. A key aspect of deployment is optimizing the code generated during the lifecycle to ensure efficient performance when live. For example, during the component update phase, understanding how to handle state props and method returns can significantly enhance the app’s responsiveness and reliability.
Ensuring each method is properly implemented allows for a solid component behavior. For example, knowing how a component behaves during the component update phase is essential when establishing communication with external data sources.
Understanding the lifecycle of a component plays a key role in both connection and deployment. By mastering these elements, constructor methods, state prop management, and efficient handling of method return values, your team can ensure applications are robust, scalable, and ready for real-world challenges.
React Component Lifecycle Examples
Understanding abstract concepts like lifecycle methods can be easier with relatable examples. Let's explore how these methods impact the behavior of a simple component.
Component mounting phase
When a component is being created and inserted into the DOM, the constructor method is the first lifecycle method called. It initializes the component's state and binds methods.
For example, in the constructor method, you can set up initial state values and bind event handlers. Following this, the static getDerivedStateFromProps method is called to sync the state with the initial props. This lifecycle method is used to update the state based on changes in props. It’s called before rendering and is crucial during the mounting phase and updating phase as well. As an example, if a child component’s state needs to be derived from its props, this method ensures the state is updated accordingly.
Component updating phase
When a component’s state or props change, the component update phase begins. The shouldComponentUpdate method is called to determine if the component should re-render.
This is followed by the method named render, which is a pure method, meaning it doesn’t modify state or props. The render method’s primary role is to return the JSX that makes up the component’s UI. And if the component contains child components, their lifecycle methods are also triggered during this phase.
Finally, the ComponentDidUpdate method is called after the component is re-rendered due to changes in state or props, and it’s useful for performing operations that depend on the updated DOM.
Component unmounting phase
When a component is removed from the DOM, the componentWillUnmount method is called. This method is called just before the component is removed from the DOM. It’s ideal for cleanup activities, such as canceling network requests or removing event listeners.
The Power of Planning to Master the React Component Lifecycle
In conclusion, React component lifecycles help development teams create dynamic and responsive web applications. By strategically utilizing methods, developers ensure components react to changes in data or user interactions without friction. That’s why when you invest in the right expertise and development best practices, your business can leverage the full potential of this JavaScript library, transforming the way your users interact with your digital presence.
Contact us today and explore which Jalasoft solution best fits your business needs!